Note
Go to the end to download the full example code
Twin evaluation of a 3D field ROM co-simulation with an FEA model#
This example shows how PyTwin can be used to load and evaluate a twin model to predict CFD results in the form of temperature fields. Temperature fields are used as inputs for an FEA thermal structural analysis of a T-junction that considers the mixing of two different flow temperatures. The example uses PyTwin to evaluate the twin results and convert them to an appropriate format. It then uses PyMAPDL to load the FEA model, apply the temperature loads coming from the twin, and perform the thermal structural analysis.
Note
To generate snapshot files at initialization time, the ROM included in the twin
must have its parameter field_data_storage_period
set to 0
and its
parameter store_snapshots
set to 1
.
To generate images files at initialization time, the ROM included in the twin must
have the Embed Geometry and Generate Image options enabled at export time.
Additionally, its parameter viewX_storage_period
must be set to 0
.
These parameters can be defined in the Twin Builder subsheet before twin compilation or be exposed as twin parameters.
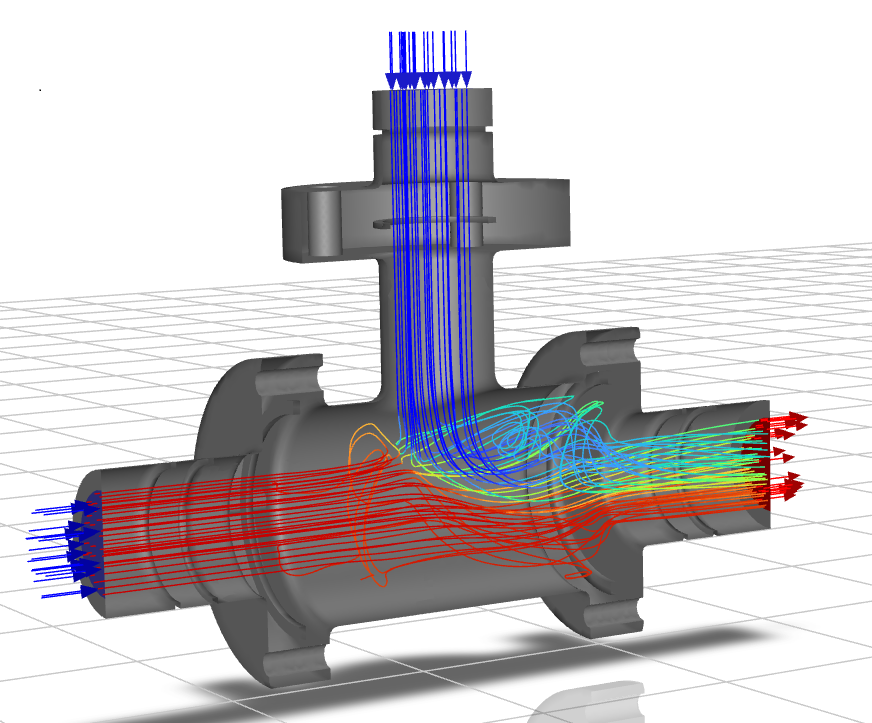
# sphinx_gallery_thumbnail_path = '_static/TBROM_cosim_pymapdl.png'
Perform required imports and launch an instance of MAPDL#
Perform required imports, which include downloading and importing the input files, and launch an instance of MAPDL.
from ansys.mapdl.core import launch_mapdl
import numpy as np
import pandas as pd
from pytwin import TwinModel, download_file
import pyvista as pv
twin_file = download_file("ThermalTBROM_23R1_other.twin", "twin_files", force_download=True)
fea_file = download_file("ThermalTBROM.dat", "other_files", force_download=True)
# start mapdl
mapdl = launch_mapdl()
print(mapdl)
PyMAPDL is taking longer than expected to connect to an MAPDL session.
Checking if there are any available licenses...
Product: Ansys Mechanical Enterprise
MAPDL Version: 22.2
ansys.mapdl Version: 0.64.0
Define inputs#
Define inputs.
cfd_inputs = {"main_inlet_temperature": 353.15, "side_inlet_temperature": 293.15}
rom_parameters = {"ThermalROM23R1_1_store_snapshots": 1}
Define auxiliary functions#
Define an auxiliary function for converting the ROM snapshot for data mapping on an FEA mesh.
def snapshot_to_fea(snapshot_file, geometry_file):
"""Create a Pandas dataframe containing the x, y, z coordinates for the ROM
and snapshot file results."""
geometry_data = np.fromfile(geometry_file, dtype=np.double, offset=8).reshape(-1, 3)
snapshot_data = np.fromfile(snapshot_file, dtype=np.double, offset=8).reshape(-1, 1)
res_list = np.hstack((geometry_data, snapshot_data))
return pd.DataFrame(res_list)
Import and save the mesh#
Reset MAPDL and import the geometry.
ANSYS Mesh
Number of Nodes: 30685
Number of Elements: 20256
Number of Element Types: 143
Number of Node Components: 0
Number of Element Components: 0
Load the twin runtime and generate temperature results#
Load the twin runtime and generate temperature results for the FEA mesh.
print("Loading model: {}".format(twin_file))
twin_model = TwinModel(twin_file)
twin_model.initialize_evaluation(inputs=cfd_inputs, parameters=rom_parameters)
rom_name = twin_model.tbrom_names[0]
snapshot = twin_model.get_snapshot_filepath(rom_name=rom_name)
geometry = twin_model.get_geometry_filepath(rom_name=rom_name)
temperature_file = snapshot_to_fea(snapshot, geometry)
Loading model: C:\Users\ansys\AppData\Local\Temp\TwinExamples\twin_files\ThermalTBROM_23R1_other.twin
Map temperature data to FEA mesh#
Map the temperature data to the FEA mesh.
temperature_data = temperature_file.values # Save data to a NumPy array
nd_temp_data = temperature_data[:, :].astype(float) # Change data type to float
# Map temperature data to the FE mesh
# Convert imported data into PolyData format
wrapped = pv.PolyData(nd_temp_data[:, :3]) # Convert NumPy array to PolyData format
wrapped["temperature"] = nd_temp_data[:, 3] # Add a scalar variable 'temperature' to PolyData
# Perform data mapping
inter_grid = grid.interpolate(
wrapped, sharpness=5, radius=0.0001, strategy="closest_point", progress_bar=True
) # Map the imported data to MAPDL grid
inter_grid.plot(show_edges=False) # Plot the interpolated data on MAPDL grid
temperature_load_val = pv.convert_array(
pv.convert_array(inter_grid.active_scalars)
) # Save temperatures interpolated to each node as a NumPy array
node_num = inter_grid.point_data["ansys_node_num"] # Save node numbers as a NumPy array
0%| [00:00<?]
Interpolating: 0%| [00:00<?]
Interpolating: 100%|##########[00:00<00:00]
Interpolating: 100%|##########[00:00<00:00]
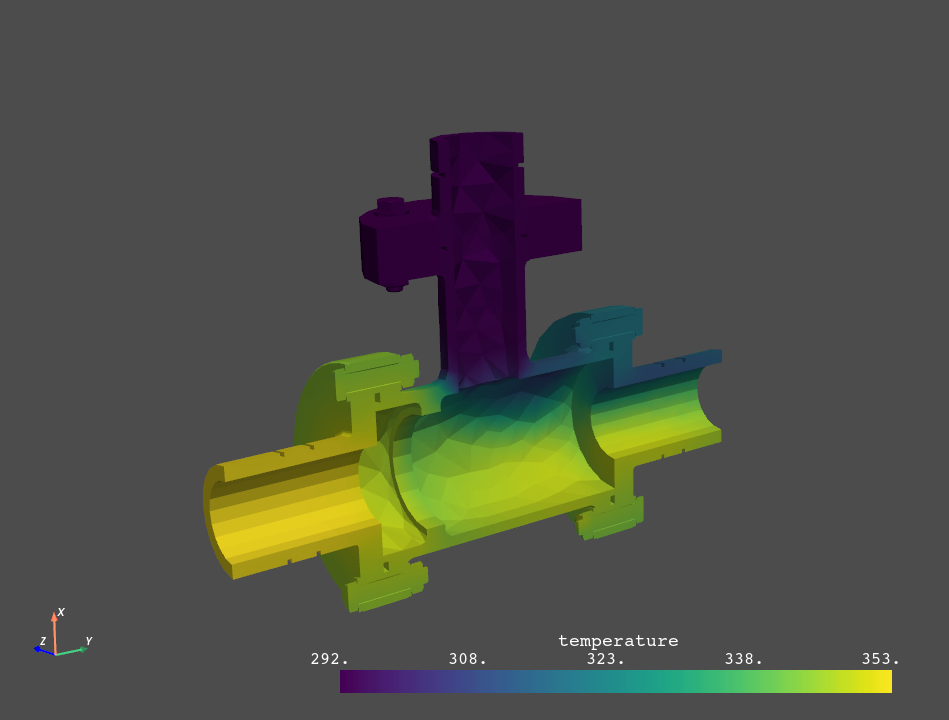
Apply loads and boundary conditions and solve the model#
Apply loads and boundary conditions and then solve the model.
# Read all nodal coordinates to an array and extract the X and Y
# minimum bounds
array_nodes = mapdl.mesh.nodes
Xmax = np.amax(array_nodes[:, 0])
Ymin = np.amin(array_nodes[:, 1])
Ymax = np.amax(array_nodes[:, 1])
# Enter /SOLU processor to apply loads and boundary conditions
mapdl.finish()
mapdl.slashsolu()
# Enter non-interactive mode to assign thermal load at each node using imported data
with mapdl.non_interactive:
for node, temp in zip(node_num, temperature_load_val):
mapdl.bf(node, "TEMP", temp)
# Use the X and Y minimum bounds to select nodes from five surfaces that are to be fixed
# Create a component and fix all degrees of freedom (DoFs)
mapdl.nsel("s", "LOC", "X", Xmax) # Select all nodes whose X coord.=Xmax
mapdl.nsel("a", "LOC", "Y", Ymin) # Select all nodes whose Y coord.=Ymin and add to previous selection
mapdl.nsel("a", "LOC", "Y", Ymax) # Select all nodes whose Y coord.=Ymax and add to previous selection
mapdl.cm("fixed_nodes", "NODE") # Create a nodal component 'fixed_nodes'
mapdl.allsel() # Revert active selection to full model
mapdl.d("fixed_nodes", "all", 0) # Impose fully fixed constraint on component created earlier
# Solve the model
output = mapdl.solve()
print(output)
*** NOTE *** CP = 16.656 TIME= 09:30:01
The automatic domain decomposition logic has selected the MESH domain
decomposition method with 2 processes per solution.
***** MAPDL SOLVE COMMAND *****
*** WARNING *** CP = 16.656 TIME= 09:30:01
Element shape checking is currently inactive. Issue SHPP,ON or
SHPP,WARN to reactivate, if desired.
*** WARNING *** CP = 16.688 TIME= 09:30:01
It has been detected that you are using reduced integration brick
elements along with the PCG solver (EQSLV,PCG). Please verify that
you have multiple elements through the thickness in your model or
switch to the SPARSE solver (EQSLV,SPARSE).
*** NOTE *** CP = 16.750 TIME= 09:30:01
The model data was checked and warning messages were found.
Please review output or errors file (
C:\Users\ansys\AppData\Local\Temp\ansys_uybkhrlytg\file0.err ) for
these warning messages.
*** SELECTION OF ELEMENT TECHNOLOGIES FOR APPLICABLE ELEMENTS ***
--- GIVE SUGGESTIONS AND RESET THE KEY OPTIONS ---
ELEMENT TYPE 1 IS SOLID187. IT IS NOT ASSOCIATED WITH FULLY INCOMPRESSIBLE
HYPERELASTIC MATERIALS. NO SUGGESTION IS AVAILABLE AND NO RESETTING IS NEEDED.
ELEMENT TYPE 2 IS SOLID187. IT IS NOT ASSOCIATED WITH FULLY INCOMPRESSIBLE
HYPERELASTIC MATERIALS. NO SUGGESTION IS AVAILABLE AND NO RESETTING IS NEEDED.
ELEMENT TYPE 3 IS SOLID187. IT IS NOT ASSOCIATED WITH FULLY INCOMPRESSIBLE
HYPERELASTIC MATERIALS. NO SUGGESTION IS AVAILABLE AND NO RESETTING IS NEEDED.
ELEMENT TYPE 4 IS SOLID186. KEYOPT(2) IS ALREADY SET AS SUGGESTED AND NO
RESETTING IS NEEDED.
ELEMENT TYPE 5 IS SOLID187. IT IS NOT ASSOCIATED WITH FULLY INCOMPRESSIBLE
HYPERELASTIC MATERIALS. NO SUGGESTION IS AVAILABLE AND NO RESETTING IS NEEDED.
ELEMENT TYPE 6 IS SOLID187. IT IS NOT ASSOCIATED WITH FULLY INCOMPRESSIBLE
HYPERELASTIC MATERIALS. NO SUGGESTION IS AVAILABLE AND NO RESETTING IS NEEDED.
ELEMENT TYPE 7 IS SOLID187. IT IS NOT ASSOCIATED WITH FULLY INCOMPRESSIBLE
HYPERELASTIC MATERIALS. NO SUGGESTION IS AVAILABLE AND NO RESETTING IS NEEDED.
ELEMENT TYPE 8 IS SOLID187. IT IS NOT ASSOCIATED WITH FULLY INCOMPRESSIBLE
HYPERELASTIC MATERIALS. NO SUGGESTION IS AVAILABLE AND NO RESETTING IS NEEDED.
ELEMENT TYPE 9 IS SOLID186. KEYOPT(2) IS ALREADY SET AS SUGGESTED AND NO
RESETTING IS NEEDED.
ELEMENT TYPE 10 IS SOLID187. IT IS NOT ASSOCIATED WITH FULLY INCOMPRESSIBLE
HYPERELASTIC MATERIALS. NO SUGGESTION IS AVAILABLE AND NO RESETTING IS NEEDED.
ELEMENT TYPE 11 IS SOLID186. KEYOPT(2) IS ALREADY SET AS SUGGESTED AND NO
RESETTING IS NEEDED.
ELEMENT TYPE 12 IS SOLID187. IT IS NOT ASSOCIATED WITH FULLY INCOMPRESSIBLE
HYPERELASTIC MATERIALS. NO SUGGESTION IS AVAILABLE AND NO RESETTING IS NEEDED.
ELEMENT TYPE 13 IS SOLID186. KEYOPT(2) IS ALREADY SET AS SUGGESTED AND NO
RESETTING IS NEEDED.
ELEMENT TYPE 14 IS SOLID187. IT IS NOT ASSOCIATED WITH FULLY INCOMPRESSIBLE
HYPERELASTIC MATERIALS. NO SUGGESTION IS AVAILABLE AND NO RESETTING IS NEEDED.
ELEMENT TYPE 15 IS SOLID186. KEYOPT(2) IS ALREADY SET AS SUGGESTED AND NO
RESETTING IS NEEDED.
ELEMENT TYPE 16 IS SOLID187. IT IS NOT ASSOCIATED WITH FULLY INCOMPRESSIBLE
HYPERELASTIC MATERIALS. NO SUGGESTION IS AVAILABLE AND NO RESETTING IS NEEDED.
ELEMENT TYPE 17 IS SOLID186. KEYOPT(2) IS ALREADY SET AS SUGGESTED AND NO
RESETTING IS NEEDED.
ELEMENT TYPE 18 IS SOLID187. IT IS NOT ASSOCIATED WITH FULLY INCOMPRESSIBLE
HYPERELASTIC MATERIALS. NO SUGGESTION IS AVAILABLE AND NO RESETTING IS NEEDED.
ELEMENT TYPE 19 IS SOLID186. KEYOPT(2) IS ALREADY SET AS SUGGESTED AND NO
RESETTING IS NEEDED.
ELEMENT TYPE 20 IS SOLID187. IT IS NOT ASSOCIATED WITH FULLY INCOMPRESSIBLE
HYPERELASTIC MATERIALS. NO SUGGESTION IS AVAILABLE AND NO RESETTING IS NEEDED.
ELEMENT TYPE 21 IS SOLID186. KEYOPT(2) IS ALREADY SET AS SUGGESTED AND NO
RESETTING IS NEEDED.
ELEMENT TYPE 22 IS SOLID187. IT IS NOT ASSOCIATED WITH FULLY INCOMPRESSIBLE
HYPERELASTIC MATERIALS. NO SUGGESTION IS AVAILABLE AND NO RESETTING IS NEEDED.
ELEMENT TYPE 23 IS SOLID186. KEYOPT(2) IS ALREADY SET AS SUGGESTED AND NO
RESETTING IS NEEDED.
ELEMENT TYPE 24 IS SOLID187. IT IS NOT ASSOCIATED WITH FULLY INCOMPRESSIBLE
HYPERELASTIC MATERIALS. NO SUGGESTION IS AVAILABLE AND NO RESETTING IS NEEDED.
ELEMENT TYPE 25 IS SOLID187. IT IS NOT ASSOCIATED WITH FULLY INCOMPRESSIBLE
HYPERELASTIC MATERIALS. NO SUGGESTION IS AVAILABLE AND NO RESETTING IS NEEDED.
ELEMENT TYPE 26 IS SOLID187. IT IS NOT ASSOCIATED WITH FULLY INCOMPRESSIBLE
HYPERELASTIC MATERIALS. NO SUGGESTION IS AVAILABLE AND NO RESETTING IS NEEDED.
ELEMENT TYPE 27 IS SOLID187. IT IS NOT ASSOCIATED WITH FULLY INCOMPRESSIBLE
HYPERELASTIC MATERIALS. NO SUGGESTION IS AVAILABLE AND NO RESETTING IS NEEDED.
ELEMENT TYPE 28 IS SOLID187. IT IS NOT ASSOCIATED WITH FULLY INCOMPRESSIBLE
HYPERELASTIC MATERIALS. NO SUGGESTION IS AVAILABLE AND NO RESETTING IS NEEDED.
ELEMENT TYPE 29 IS SOLID187. IT IS NOT ASSOCIATED WITH FULLY INCOMPRESSIBLE
HYPERELASTIC MATERIALS. NO SUGGESTION IS AVAILABLE AND NO RESETTING IS NEEDED.
ELEMENT TYPE 30 IS SOLID187. IT IS NOT ASSOCIATED WITH FULLY INCOMPRESSIBLE
HYPERELASTIC MATERIALS. NO SUGGESTION IS AVAILABLE AND NO RESETTING IS NEEDED.
ELEMENT TYPE 31 IS SOLID187. IT IS NOT ASSOCIATED WITH FULLY INCOMPRESSIBLE
HYPERELASTIC MATERIALS. NO SUGGESTION IS AVAILABLE AND NO RESETTING IS NEEDED.
ELEMENT TYPE 32 IS SOLID187. IT IS NOT ASSOCIATED WITH FULLY INCOMPRESSIBLE
HYPERELASTIC MATERIALS. NO SUGGESTION IS AVAILABLE AND NO RESETTING IS NEEDED.
ELEMENT TYPE 33 IS SOLID186. KEYOPT(2) IS ALREADY SET AS SUGGESTED AND NO
RESETTING IS NEEDED.
ELEMENT TYPE 34 IS SOLID187. IT IS NOT ASSOCIATED WITH FULLY INCOMPRESSIBLE
HYPERELASTIC MATERIALS. NO SUGGESTION IS AVAILABLE AND NO RESETTING IS NEEDED.
ELEMENT TYPE 35 IS SOLID186. KEYOPT(2) IS ALREADY SET AS SUGGESTED AND NO
RESETTING IS NEEDED.
ELEMENT TYPE 36 IS SOLID187. IT IS NOT ASSOCIATED WITH FULLY INCOMPRESSIBLE
HYPERELASTIC MATERIALS. NO SUGGESTION IS AVAILABLE AND NO RESETTING IS NEEDED.
ELEMENT TYPE 37 IS SOLID186. KEYOPT(2) IS ALREADY SET AS SUGGESTED AND NO
RESETTING IS NEEDED.
ELEMENT TYPE 38 IS SOLID186. KEYOPT(2) IS ALREADY SET AS SUGGESTED AND NO
RESETTING IS NEEDED.
ELEMENT TYPE 39 IS SOLID187. IT IS NOT ASSOCIATED WITH FULLY INCOMPRESSIBLE
HYPERELASTIC MATERIALS. NO SUGGESTION IS AVAILABLE AND NO RESETTING IS NEEDED.
ELEMENT TYPE 40 IS SOLID186. KEYOPT(2) IS ALREADY SET AS SUGGESTED AND NO
RESETTING IS NEEDED.
ELEMENT TYPE 41 IS SOLID187. IT IS NOT ASSOCIATED WITH FULLY INCOMPRESSIBLE
HYPERELASTIC MATERIALS. NO SUGGESTION IS AVAILABLE AND NO RESETTING IS NEEDED.
ELEMENT TYPE 42 IS SOLID186. KEYOPT(2) IS ALREADY SET AS SUGGESTED AND NO
RESETTING IS NEEDED.
ELEMENT TYPE 43 IS SOLID186. KEYOPT(2) IS ALREADY SET AS SUGGESTED AND NO
RESETTING IS NEEDED.
ELEMENT TYPE 44 IS SOLID187. IT IS NOT ASSOCIATED WITH FULLY INCOMPRESSIBLE
HYPERELASTIC MATERIALS. NO SUGGESTION IS AVAILABLE AND NO RESETTING IS NEEDED.
ELEMENT TYPE 45 IS SOLID187. IT IS NOT ASSOCIATED WITH FULLY INCOMPRESSIBLE
HYPERELASTIC MATERIALS. NO SUGGESTION IS AVAILABLE AND NO RESETTING IS NEEDED.
ELEMENT TYPE 46 IS SOLID187. IT IS NOT ASSOCIATED WITH FULLY INCOMPRESSIBLE
HYPERELASTIC MATERIALS. NO SUGGESTION IS AVAILABLE AND NO RESETTING IS NEEDED.
ELEMENT TYPE 47 IS SOLID187. IT IS NOT ASSOCIATED WITH FULLY INCOMPRESSIBLE
HYPERELASTIC MATERIALS. NO SUGGESTION IS AVAILABLE AND NO RESETTING IS NEEDED.
ELEMENT TYPE 48 IS SOLID187. IT IS NOT ASSOCIATED WITH FULLY INCOMPRESSIBLE
HYPERELASTIC MATERIALS. NO SUGGESTION IS AVAILABLE AND NO RESETTING IS NEEDED.
ELEMENT TYPE 49 IS SOLID187. IT IS NOT ASSOCIATED WITH FULLY INCOMPRESSIBLE
HYPERELASTIC MATERIALS. NO SUGGESTION IS AVAILABLE AND NO RESETTING IS NEEDED.
ELEMENT TYPE 50 IS SOLID186. KEYOPT(2) IS ALREADY SET AS SUGGESTED AND NO
RESETTING IS NEEDED.
ELEMENT TYPE 51 IS SOLID187. IT IS NOT ASSOCIATED WITH FULLY INCOMPRESSIBLE
HYPERELASTIC MATERIALS. NO SUGGESTION IS AVAILABLE AND NO RESETTING IS NEEDED.
ELEMENT TYPE 52 IS SOLID186. KEYOPT(2) IS ALREADY SET AS SUGGESTED AND NO
RESETTING IS NEEDED.
ELEMENT TYPE 53 IS SOLID186. KEYOPT(2) IS ALREADY SET AS SUGGESTED AND NO
RESETTING IS NEEDED.
ELEMENT TYPE 54 IS SOLID186. KEYOPT(2) IS ALREADY SET AS SUGGESTED AND NO
RESETTING IS NEEDED.
*** MAPDL - ENGINEERING ANALYSIS SYSTEM RELEASE 2022 R2 22.2 ***
Ansys Mechanical Enterprise
00000000 VERSION=WINDOWS x64 09:30:01 DEC 14, 2023 CP= 16.766
T_Junction_1way_steady-state--Static Structural (B5)
S O L U T I O N O P T I O N S
PROBLEM DIMENSIONALITY. . . . . . . . . . . . .3-D
DEGREES OF FREEDOM. . . . . . UX UY UZ
ANALYSIS TYPE . . . . . . . . . . . . . . . . .STATIC (STEADY-STATE)
OFFSET TEMPERATURE FROM ABSOLUTE ZERO . . . . . 273.15
EQUATION SOLVER OPTION. . . . . . . . . . . . .PCG
TOLERANCE. . . . . . . . . . . . . . . . . . 1.00000E-08
GLOBALLY ASSEMBLED MATRIX . . . . . . . . . . .SYMMETRIC
*** WARNING *** CP = 16.781 TIME= 09:30:01
Material number 143 (used by element 29444) should normally have at
least one MP or one TB type command associated with it. Output of
energy by material may not be available.
*** NOTE *** CP = 16.797 TIME= 09:30:01
The step data was checked and warning messages were found.
Please review output or errors file (
C:\Users\ansys\AppData\Local\Temp\ansys_uybkhrlytg\file0.err ) for
these warning messages.
*** NOTE *** CP = 16.797 TIME= 09:30:01
The conditions for direct assembly have been met. No .emat or .erot
files will be produced.
*** NOTE *** CP = 17.141 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 55 and contact element type 55 has been set up. The
companion pair has real constant set ID 56. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.17596E+17
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.22732E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.31042E-02
Average contact pair depth 0.22732E-02
Average target surface length 0.30935E-02
Default pinball region factor PINB 0.25000
*WARNING*: The default pinball radius may be too small to capture
contacting zone under small sliding assumption. Redefine the pinball
radius if necessary.
The resulting pinball region 0.56830E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.141 TIME= 09:30:01
Min. Initial gap 4.892041643E-04 was detected between contact element
24597 and target element 24797.
Contact is detected due to initial settings.
Max. Geometric gap 5.108346823E-04 has been detected between contact
element 24608 and target element 24805.
*WARNING*: The geometric gap/penetration may be too large. Increase
pinball radius if it is a true geometric gap/penetration. Decrease
pinball if it is a false one.
****************************************
*** NOTE *** CP = 17.141 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 56 and contact element type 55 has been set up. The
companion pair has real constant set ID 55. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.17596E+17
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.22155E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.32205E-02
Average contact pair depth 0.22155E-02
Average target surface length 0.29897E-02
Default pinball region factor PINB 0.25000
*WARNING*: The default pinball radius may be too small to capture
contacting zone under small sliding assumption. Redefine the pinball
radius if necessary.
The resulting pinball region 0.55388E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.141 TIME= 09:30:01
Min. Initial gap 4.914598591E-04 was detected between contact element
24703 and target element 24481.
Contact is detected due to initial settings.
Max. Geometric gap 5.103830541E-04 has been detected between contact
element 24711 and target element 24504.
*WARNING*: The geometric gap/penetration may be too large. Increase
pinball radius if it is a true geometric gap/penetration. Decrease
pinball if it is a false one.
****************************************
*** NOTE *** CP = 17.141 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 57 and contact element type 57 has been set up. The
companion pair has real constant set ID 58. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.10201E+17
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.39212E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.46042E-02
Average contact pair depth 0.39212E-02
Average target surface length 0.28864E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.98030E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.141 TIME= 09:30:01
Min. Initial gap 4.910065956E-04 was detected between contact element
24874 and target element 24952.
Contact is detected due to initial settings.
Max. Geometric gap 5.061191669E-04 has been detected between contact
element 24876 and target element 24950.
*WARNING*: The geometric gap/penetration may be too large. Increase
pinball radius if it is a true geometric gap/penetration. Decrease
pinball if it is a false one.
****************************************
*** NOTE *** CP = 17.141 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 58 and contact element type 57 has been set up. The
companion pair has real constant set ID 57. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.10201E+17
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.25334E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.29829E-02
Average contact pair depth 0.25334E-02
Average target surface length 0.44708E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.63336E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.141 TIME= 09:30:01
Min. Initial gap 4.920431168E-04 was detected between contact element
24917 and target element 24833.
Contact is detected due to initial settings.
Max. Geometric gap 5.061138968E-04 has been detected between contact
element 24909 and target element 24836.
*WARNING*: The geometric gap/penetration may be too large. Increase
pinball radius if it is a true geometric gap/penetration. Decrease
pinball if it is a false one.
****************************************
*** NOTE *** CP = 17.141 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 59 and contact element type 59 has been set up. The
companion pair has real constant set ID 60. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.60075E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.44676E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.59960E-02
Average contact pair depth 0.44676E-02
Average target surface length 0.14709E-01
Default pinball region factor PINB 0.25000
*WARNING*: The default pinball radius may be too small to capture
contacting zone under small sliding assumption. Redefine the pinball
radius if necessary.
The resulting pinball region 0.11169E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.141 TIME= 09:30:01
Min. Initial gap 3.496108984E-04 was detected between contact element
25077 and target element 25110.
Contact is detected due to initial settings.
Max. Geometric gap 1.116099622E-03 has been detected between contact
element 25085 and target element 25106.
*WARNING*: The geometric gap/penetration may be too large. Increase
pinball radius if it is a true geometric gap/penetration. Decrease
pinball if it is a false one.
****************************************
*** NOTE *** CP = 17.141 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 60 and contact element type 59 has been set up. The
companion pair has real constant set ID 59. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.60075E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.66583E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.15831E-01
Average contact pair depth 0.66583E-02
Average target surface length 0.58424E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.16646E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.141 TIME= 09:30:01
Min. Initial gap 3.532073558E-04 was detected between contact element
25098 and target element 24995.
Contact is detected due to initial settings.
Max. Geometric gap 1.544991189E-03 has been detected between contact
element 25094 and target element 25020.
*WARNING*: The geometric gap/penetration may be too large. Increase
pinball radius if it is a true geometric gap/penetration. Decrease
pinball if it is a false one.
****************************************
*** NOTE *** CP = 17.141 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 61 and contact element type 61 has been set up. The
companion pair has real constant set ID 62. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.51152E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.33229E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.49022E-02
Average contact pair depth 0.33229E-02
Average target surface length 0.14531E-01
Default pinball region factor PINB 0.25000
*WARNING*: The default pinball radius may be too small to capture
contacting zone under small sliding assumption. Redefine the pinball
radius if necessary.
The resulting pinball region 0.83073E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.141 TIME= 09:30:01
Min. Initial gap 2.598040708E-04 was detected between contact element
25254 and target element 25302.
Contact is detected due to initial settings.
Max. Geometric gap 6.188006116E-04 has been detected between contact
element 25275 and target element 25298.
*WARNING*: The geometric gap/penetration may be too large. Increase
pinball radius if it is a true geometric gap/penetration. Decrease
pinball if it is a false one.
****************************************
*** NOTE *** CP = 17.141 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 62 and contact element type 61 has been set up. The
companion pair has real constant set ID 61. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.51152E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.78199E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.15617E-01
Average contact pair depth 0.78199E-02
Average target surface length 0.47793E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.19550E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.141 TIME= 09:30:01
Min. Initial gap 2.739881357E-04 was detected between contact element
25290 and target element 25174.
Contact is detected due to initial settings.
Max. Geometric gap 5.011163479E-04 has been detected between contact
element 25283 and target element 25162.
****************************************
*** NOTE *** CP = 17.141 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 63 and contact element type 63 has been set up. The
companion pair has real constant set ID 64. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.83686E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.18117E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.29027E-02
Average contact pair depth 0.18117E-02
Average target surface length 0.81936E-02
Default pinball region factor PINB 0.25000
*WARNING*: The default pinball radius may be too small to capture
contacting zone under small sliding assumption. Redefine the pinball
radius if necessary.
The resulting pinball region 0.45292E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.141 TIME= 09:30:01
No contact was detected for this contact pair.
****************************************
*** NOTE *** CP = 17.141 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 64 and contact element type 63 has been set up. The
companion pair has real constant set ID 63. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.83686E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.47798E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.87520E-02
Average contact pair depth 0.47798E-02
Average target surface length 0.29186E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.11949E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.141 TIME= 09:30:01
Min. Initial gap 5.008751444E-04 was detected between contact element
25327 and target element 25310.
Contact is detected due to initial settings.
Max. Geometric gap 6.116710797E-04 has been detected between contact
element 25330 and target element 25313.
*WARNING*: The geometric gap/penetration may be too large. Increase
pinball radius if it is a true geometric gap/penetration. Decrease
pinball if it is a false one.
****************************************
*** NOTE *** CP = 17.141 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 65 and contact element type 65 has been set up. The
companion pair has real constant set ID 66. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.47822E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.22750E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.36454E-02
Average contact pair depth 0.22750E-02
Average target surface length 0.98585E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.56876E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.141 TIME= 09:30:01
Max. Initial penetration 1.736955052E-04 was detected between contact
element 25361 and target element 25388.
You may move entire target surface by : x= 2.91539766E-20, y=
-1.736955052E-04, z= -3.343422002E-20,to reduce initial penetration.
****************************************
*** NOTE *** CP = 17.141 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 66 and contact element type 65 has been set up. The
companion pair has real constant set ID 65. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.47822E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.83643E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.10201E-01
Average contact pair depth 0.83643E-02
Average target surface length 0.37691E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.20911E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.141 TIME= 09:30:01
Max. Initial penetration 6.195620121E-05 was detected between contact
element 25372 and target element 25349.
You may move entire target surface by : x= -8.98413284E-06, y=
6.129957E-05, z= 4.679138581E-07,to reduce initial penetration.
****************************************
*** NOTE *** CP = 17.141 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 67 and contact element type 67 has been set up. The
companion pair has real constant set ID 68. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.67332E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.24430E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.67716E-02
Average contact pair depth 0.24430E-02
Average target surface length 0.13778E-01
Default pinball region factor PINB 0.25000
The resulting pinball region 0.61076E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.141 TIME= 09:30:01
Max. Initial penetration 2.775557562E-17 was detected between contact
element 25423 and target element 25459.
****************************************
*** NOTE *** CP = 17.141 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 68 and contact element type 67 has been set up. The
companion pair has real constant set ID 67. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.67332E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.59407E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.14115E-01
Average contact pair depth 0.59407E-02
Average target surface length 0.67254E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.14852E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.141 TIME= 09:30:01
Max. Initial penetration 2.775557562E-17 was detected between contact
element 25439 and target element 25413.
****************************************
*** NOTE *** CP = 17.141 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 69 and contact element type 69 has been set up. The
companion pair has real constant set ID 70. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.37743E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.24103E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.67716E-02
Average contact pair depth 0.24103E-02
Average target surface length 0.12921E-01
Default pinball region factor PINB 0.25000
The resulting pinball region 0.60258E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.141 TIME= 09:30:01
Max. Initial penetration 1.021405789E-04 was detected between contact
element 25492 and target element 25546.
You may move entire target surface by : x= 3.178767662E-19, y=
1.021405789E-04, z= -4.486295031E-19,to reduce initial penetration.
****************************************
*** NOTE *** CP = 17.141 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 70 and contact element type 69 has been set up. The
companion pair has real constant set ID 69. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.37743E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.10598E-02
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.13550E-01
Average contact pair depth 0.10598E-01
Average target surface length 0.67254E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.26495E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.141 TIME= 09:30:01
Max. Initial penetration 7.29363013E-05 was detected between contact
element 25520 and target element 25475.
You may move entire target surface by : x= -4.791479912E-07, y=
-7.201771111E-05, z= 1.152925631E-05,to reduce initial penetration.
****************************************
*** NOTE *** CP = 17.141 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 71 and contact element type 71 has been set up. The
companion pair has real constant set ID 72. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.82245E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.15807E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.29429E-02
Average contact pair depth 0.15807E-02
Average target surface length 0.89008E-02
Default pinball region factor PINB 0.25000
*WARNING*: The default pinball radius may be too small to capture
contacting zone under small sliding assumption. Redefine the pinball
radius if necessary.
The resulting pinball region 0.39518E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.141 TIME= 09:30:01
No contact was detected for this contact pair.
****************************************
*** NOTE *** CP = 17.141 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 72 and contact element type 71 has been set up. The
companion pair has real constant set ID 71. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.82245E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.48635E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.95806E-02
Average contact pair depth 0.48635E-02
Average target surface length 0.29151E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.12159E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.141 TIME= 09:30:01
Min. Initial gap 4.996627913E-04 was detected between contact element
25591 and target element 25558.
Contact is detected due to initial settings.
Max. Geometric gap 8.477829511E-04 has been detected between contact
element 25580 and target element 25566.
*WARNING*: The geometric gap/penetration may be too large. Increase
pinball radius if it is a true geometric gap/penetration. Decrease
pinball if it is a false one.
****************************************
*** NOTE *** CP = 17.141 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 73 and contact element type 73 has been set up. The
companion pair has real constant set ID 74. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.48536E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.21852E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.36454E-02
Average contact pair depth 0.21852E-02
Average target surface length 0.96458E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.54630E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.141 TIME= 09:30:01
Max. Initial penetration 1.736954878E-04 was detected between contact
element 25627 and target element 25656.
You may move entire target surface by : x= -1.162760459E-18, y=
1.736954878E-04, z= 1.256153359E-18,to reduce initial penetration.
****************************************
*** NOTE *** CP = 17.141 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 74 and contact element type 73 has been set up. The
companion pair has real constant set ID 73. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.48536E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.82412E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.99777E-02
Average contact pair depth 0.82412E-02
Average target surface length 0.37691E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.20603E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.141 TIME= 09:30:01
Max. Initial penetration 6.195620923E-05 was detected between contact
element 25639 and target element 25615.
You may move entire target surface by : x= -8.984135126E-06, y=
-6.129957778E-05, z= 4.679135334E-07,to reduce initial penetration.
****************************************
*** NOTE *** CP = 17.141 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 75 and contact element type 75 has been set up. The
companion pair has real constant set ID 76. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.10596E+17
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.37750E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.57784E-02
Average contact pair depth 0.37750E-02
Average target surface length 0.35009E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.94375E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.141 TIME= 09:30:01
Min. Initial gap 4.990860284E-04 was detected between contact element
25711 and target element 25754.
Contact is detected due to initial settings.
Max. Geometric gap 6.638924593E-04 has been detected between contact
element 25716 and target element 25755.
*WARNING*: The geometric gap/penetration may be too large. Increase
pinball radius if it is a true geometric gap/penetration. Decrease
pinball if it is a false one.
****************************************
*** NOTE *** CP = 17.141 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 76 and contact element type 75 has been set up. The
companion pair has real constant set ID 75. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.10596E+17
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.19156E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.34841E-02
Average contact pair depth 0.19156E-02
Average target surface length 0.53755E-02
Default pinball region factor PINB 0.25000
*WARNING*: The default pinball radius may be too small to capture
contacting zone under small sliding assumption. Redefine the pinball
radius if necessary.
The resulting pinball region 0.47891E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.141 TIME= 09:30:01
No contact was detected for this contact pair.
****************************************
*** NOTE *** CP = 17.141 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 77 and contact element type 77 has been set up. The
companion pair has real constant set ID 78. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.88335E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.45282E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.79821E-02
Average contact pair depth 0.45282E-02
Average target surface length 0.29186E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.11321E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.141 TIME= 09:30:01
Min. Initial gap 4.991211658E-04 was detected between contact element
25776 and target element 25808.
Contact is detected due to initial settings.
Max. Geometric gap 5.769895291E-04 has been detected between contact
element 25780 and target element 25809.
*WARNING*: The geometric gap/penetration may be too large. Increase
pinball radius if it is a true geometric gap/penetration. Decrease
pinball if it is a false one.
****************************************
*** NOTE *** CP = 17.141 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 78 and contact element type 77 has been set up. The
companion pair has real constant set ID 77. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.88335E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.18117E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.29027E-02
Average contact pair depth 0.18117E-02
Average target surface length 0.75160E-02
Default pinball region factor PINB 0.25000
*WARNING*: The default pinball radius may be too small to capture
contacting zone under small sliding assumption. Redefine the pinball
radius if necessary.
The resulting pinball region 0.45292E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.141 TIME= 09:30:01
No contact was detected for this contact pair.
****************************************
*** NOTE *** CP = 17.141 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 79 and contact element type 79 has been set up. The
companion pair has real constant set ID 80. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.59545E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.56296E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.88012E-02
Average contact pair depth 0.56296E-02
Average target surface length 0.86839E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.14074E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.141 TIME= 09:30:01
Min. Initial gap 4.350596782E-04 was detected between contact element
25846 and target element 25866.
Contact is detected due to initial settings.
Max. Geometric gap 4.922200165E-04 has been detected between contact
element 25847 and target element 25868.
****************************************
*** NOTE *** CP = 17.141 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 80 and contact element type 79 has been set up. The
companion pair has real constant set ID 79. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.59545E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.67176E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.88969E-02
Average contact pair depth 0.67176E-02
Average target surface length 0.83043E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.16794E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.141 TIME= 09:30:01
Min. Initial gap 4.369370711E-04 was detected between contact element
25854 and target element 25821.
Contact is detected due to initial settings.
Max. Geometric gap 4.873658423E-04 has been detected between contact
element 25863 and target element 25820.
****************************************
*** NOTE *** CP = 17.141 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 81 and contact element type 81 has been set up. The
companion pair has real constant set ID 82. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.60222E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.57913E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.98935E-02
Average contact pair depth 0.57913E-02
Average target surface length 0.86839E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.14478E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.141 TIME= 09:30:01
Min. Initial gap 4.441485883E-04 was detected between contact element
25912 and target element 25935.
Contact is detected due to initial settings.
Max. Geometric gap 5.632278433E-04 has been detected between contact
element 25913 and target element 25938.
****************************************
*** NOTE *** CP = 17.141 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 82 and contact element type 81 has been set up. The
companion pair has real constant set ID 81. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.60222E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.66421E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.88969E-02
Average contact pair depth 0.66421E-02
Average target surface length 0.92954E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.16605E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.141 TIME= 09:30:01
Min. Initial gap 4.294073687E-04 was detected between contact element
25923 and target element 25890.
Contact is detected due to initial settings.
Max. Geometric gap 6.261542594E-04 has been detected between contact
element 25925 and target element 25884.
****************************************
*** NOTE *** CP = 17.141 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 83 and contact element type 83 has been set up. The
companion pair has real constant set ID 84. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.60735E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.22691E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.36454E-02
Average contact pair depth 0.22691E-02
Average target surface length 0.11876E-01
Default pinball region factor PINB 0.25000
The resulting pinball region 0.56728E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.141 TIME= 09:30:01
Max. Initial penetration 5.551115123E-17 was detected between contact
element 25963 and target element 25989.
****************************************
*** NOTE *** CP = 17.141 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 84 and contact element type 83 has been set up. The
companion pair has real constant set ID 83. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.60735E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.65860E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.12255E-01
Average contact pair depth 0.65860E-02
Average target surface length 0.37691E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.16465E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.141 TIME= 09:30:01
Max. Initial penetration 8.326672685E-17 was detected between contact
element 25972 and target element 25946.
****************************************
*** NOTE *** CP = 17.141 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 85 and contact element type 85 has been set up. The
companion pair has real constant set ID 86. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.53384E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.22750E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.36454E-02
Average contact pair depth 0.22750E-02
Average target surface length 0.10238E-01
Default pinball region factor PINB 0.25000
The resulting pinball region 0.56876E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.141 TIME= 09:30:01
Max. Initial penetration 1.736954878E-04 was detected between contact
element 26009 and target element 26050.
You may move entire target surface by : x= 1.054076711E-18, y=
1.736954878E-04, z= 1.298304891E-18,to reduce initial penetration.
****************************************
*** NOTE *** CP = 17.141 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 86 and contact element type 85 has been set up. The
companion pair has real constant set ID 85. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.53384E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.74929E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.10632E-01
Average contact pair depth 0.74929E-02
Average target surface length 0.37691E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.18732E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.141 TIME= 09:30:01
Max. Initial penetration 6.195620924E-05 was detected between contact
element 26033 and target element 25997.
You may move entire target surface by : x= 8.984135126E-06, y=
-6.129957778E-05, z= 4.679135334E-07,to reduce initial penetration.
****************************************
*** NOTE *** CP = 17.141 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 87 and contact element type 87 has been set up. The
companion pair has real constant set ID 88. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.94595E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.42286E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.57472E-02
Average contact pair depth 0.42286E-02
Average target surface length 0.37019E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.10571E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.141 TIME= 09:30:01
Min. Initial gap 4.990834106E-04 was detected between contact element
26104 and target element 26139.
Contact is detected due to initial settings.
Max. Geometric gap 6.97250943E-04 has been detected between contact
element 26113 and target element 26137.
*WARNING*: The geometric gap/penetration may be too large. Increase
pinball radius if it is a true geometric gap/penetration. Decrease
pinball if it is a false one.
****************************************
*** NOTE *** CP = 17.141 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 88 and contact element type 87 has been set up. The
companion pair has real constant set ID 87. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.94595E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.24192E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.35975E-02
Average contact pair depth 0.24192E-02
Average target surface length 0.54068E-02
Default pinball region factor PINB 0.25000
*WARNING*: The default pinball radius may be too small to capture
contacting zone under small sliding assumption. Redefine the pinball
radius if necessary.
The resulting pinball region 0.60481E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.141 TIME= 09:30:01
Min. Initial gap 4.991700363E-04 was detected between contact element
26127 and target element 26073.
Contact is detected due to initial settings.
Max. Geometric gap 6.001E-04 has been detected between contact element
26117 and target element 26061.
*WARNING*: The geometric gap/penetration may be too large. Increase
pinball radius if it is a true geometric gap/penetration. Decrease
pinball if it is a false one.
****************************************
*** NOTE *** CP = 17.141 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 89 and contact element type 89 has been set up. The
companion pair has real constant set ID 90. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.62088E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.64425E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.10904E-01
Average contact pair depth 0.64425E-02
Average target surface length 0.91710E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.16106E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.141 TIME= 09:30:01
Min. Initial gap 4.237187747E-04 was detected between contact element
26176 and target element 26205.
Contact is detected due to initial settings.
Max. Geometric gap 5.530475212E-04 has been detected between contact
element 26162 and target element 26199.
****************************************
*** NOTE *** CP = 17.141 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 90 and contact element type 89 has been set up. The
companion pair has real constant set ID 89. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.62088E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.59953E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.94667E-02
Average contact pair depth 0.59953E-02
Average target surface length 0.10188E-01
Default pinball region factor PINB 0.25000
The resulting pinball region 0.14988E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.141 TIME= 09:30:01
Min. Initial gap 4.237389866E-04 was detected between contact element
26193 and target element 26155.
Contact is detected due to initial settings.
Max. Geometric gap 6.133708155E-04 has been detected between contact
element 26184 and target element 26141.
****************************************
*** NOTE *** CP = 17.141 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 91 and contact element type 91 has been set up. The
companion pair has real constant set ID 92. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.62448E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.64053E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.97988E-02
Average contact pair depth 0.64053E-02
Average target surface length 0.88085E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.16013E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.141 TIME= 09:30:01
Min. Initial gap 4.34010795E-04 was detected between contact element
26236 and target element 26276.
Contact is detected due to initial settings.
Max. Geometric gap 1.432523123E-03 has been detected between contact
element 26248 and target element 26268.
*WARNING*: The geometric gap/penetration may be too large. Increase
pinball radius if it is a true geometric gap/penetration. Decrease
pinball if it is a false one.
****************************************
*** NOTE *** CP = 17.141 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 92 and contact element type 91 has been set up. The
companion pair has real constant set ID 91. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.62448E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.60337E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.90933E-02
Average contact pair depth 0.60337E-02
Average target surface length 0.92820E-02
Default pinball region factor PINB 0.25000
*WARNING*: The default pinball radius may be too small to capture
contacting zone under small sliding assumption. Redefine the pinball
radius if necessary.
The resulting pinball region 0.15084E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.141 TIME= 09:30:01
Min. Initial gap 4.245125538E-04 was detected between contact element
26263 and target element 26213.
Contact is detected due to initial settings.
Max. Geometric gap 1.225092739E-03 has been detected between contact
element 26260 and target element 26225.
*WARNING*: The geometric gap/penetration may be too large. Increase
pinball radius if it is a true geometric gap/penetration. Decrease
pinball if it is a false one.
****************************************
*** NOTE *** CP = 17.141 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 93 and contact element type 93 has been set up. The
companion pair has real constant set ID 94. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.17429E+17
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.22950E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.31471E-02
Average contact pair depth 0.22950E-02
Average target surface length 0.30838E-02
Default pinball region factor PINB 0.25000
*WARNING*: The default pinball radius may be too small to capture
contacting zone under small sliding assumption. Redefine the pinball
radius if necessary.
The resulting pinball region 0.57376E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.141 TIME= 09:30:01
Min. Initial gap 4.918864337E-04 was detected between contact element
26465 and target element 26665.
Contact is detected due to initial settings.
Max. Geometric gap 5.046381826E-04 has been detected between contact
element 26460 and target element 26660.
*WARNING*: The geometric gap/penetration may be too large. Increase
pinball radius if it is a true geometric gap/penetration. Decrease
pinball if it is a false one.
****************************************
*** NOTE *** CP = 17.141 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 94 and contact element type 93 has been set up. The
companion pair has real constant set ID 93. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.17429E+17
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.22169E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.32086E-02
Average contact pair depth 0.22169E-02
Average target surface length 0.30320E-02
Default pinball region factor PINB 0.25000
*WARNING*: The default pinball radius may be too small to capture
contacting zone under small sliding assumption. Redefine the pinball
radius if necessary.
The resulting pinball region 0.55424E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.141 TIME= 09:30:01
Min. Initial gap 4.910400799E-04 was detected between contact element
26570 and target element 26362.
Contact is detected due to initial settings.
Max. Geometric gap 5.057185782E-04 has been detected between contact
element 26554 and target element 26357.
*WARNING*: The geometric gap/penetration may be too large. Increase
pinball radius if it is a true geometric gap/penetration. Decrease
pinball if it is a false one.
****************************************
*** NOTE *** CP = 17.141 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 95 and contact element type 95 has been set up. The
companion pair has real constant set ID 96. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.10125E+17
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.39504E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.50026E-02
Average contact pair depth 0.39504E-02
Average target surface length 0.28060E-02
Default pinball region factor PINB 0.25000
*WARNING*: The default pinball radius may be too small to capture
contacting zone under small sliding assumption. Redefine the pinball
radius if necessary.
The resulting pinball region 0.98761E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.141 TIME= 09:30:01
Min. Initial gap 4.957711692E-04 was detected between contact element
26733 and target element 26809.
Contact is detected due to initial settings.
Max. Geometric gap 6.258167582E-04 has been detected between contact
element 26734 and target element 26811.
*WARNING*: The geometric gap/penetration may be too large. Increase
pinball radius if it is a true geometric gap/penetration. Decrease
pinball if it is a false one.
****************************************
*** NOTE *** CP = 17.141 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 96 and contact element type 95 has been set up. The
companion pair has real constant set ID 95. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.10125E+17
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.22075E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.29125E-02
Average contact pair depth 0.22075E-02
Average target surface length 0.48301E-02
Default pinball region factor PINB 0.25000
*WARNING*: The default pinball radius may be too small to capture
contacting zone under small sliding assumption. Redefine the pinball
radius if necessary.
The resulting pinball region 0.55187E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.141 TIME= 09:30:01
Min. Initial gap 4.914825104E-04 was detected between contact element
26771 and target element 26711.
Contact is detected due to initial settings.
Max. Geometric gap 5.460017812E-04 has been detected between contact
element 26755 and target element 26687.
*WARNING*: The geometric gap/penetration may be too large. Increase
pinball radius if it is a true geometric gap/penetration. Decrease
pinball if it is a false one.
****************************************
*** NOTE *** CP = 17.141 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 97 and contact element type 97 has been set up. The
companion pair has real constant set ID 98. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.31133E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.24430E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.67716E-02
Average contact pair depth 0.24430E-02
Average target surface length 0.19342E-01
Default pinball region factor PINB 0.25000
The resulting pinball region 0.61076E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.141 TIME= 09:30:01
Max. Initial penetration 2.775557562E-17 was detected between contact
element 26842 and target element 26873.
****************************************
*** NOTE *** CP = 17.141 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 98 and contact element type 97 has been set up. The
companion pair has real constant set ID 97. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.31133E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.12848E-02
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.20130E-01
Average contact pair depth 0.12848E-01
Average target surface length 0.67254E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.32120E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.141 TIME= 09:30:01
Max. Initial penetration 2.775557562E-17 was detected between contact
element 26870 and target element 26834.
****************************************
*** NOTE *** CP = 17.141 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 99 and contact element type 99 has been set up. The
companion pair has real constant set ID 100. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.33165E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.24139E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.67716E-02
Average contact pair depth 0.24139E-02
Average target surface length 0.17168E-01
Default pinball region factor PINB 0.25000
The resulting pinball region 0.60349E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.141 TIME= 09:30:01
Max. Initial penetration 2.775557562E-17 was detected between contact
element 26908 and target element 26941.
****************************************
*** NOTE *** CP = 17.156 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 100 and contact element type 99 has been set up. The
companion pair has real constant set ID 99. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.33165E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.12061E-02
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.17767E-01
Average contact pair depth 0.12061E-01
Average target surface length 0.67254E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.30153E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.156 TIME= 09:30:01
Max. Initial penetration 0 was detected between contact element 26924
and target element 26899.
****************************************
*** NOTE *** CP = 17.156 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 101 and contact element type 101 has been set up. The
companion pair has real constant set ID 102. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.17100E+17
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.23392E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.31249E-02
Average contact pair depth 0.23392E-02
Average target surface length 0.30838E-02
Default pinball region factor PINB 0.25000
*WARNING*: The default pinball radius may be too small to capture
contacting zone under small sliding assumption. Redefine the pinball
radius if necessary.
The resulting pinball region 0.58479E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.156 TIME= 09:30:01
Min. Initial gap 4.918864151E-04 was detected between contact element
27140 and target element 27339.
Contact is detected due to initial settings.
Max. Geometric gap 5.046381865E-04 has been detected between contact
element 27135 and target element 27334.
*WARNING*: The geometric gap/penetration may be too large. Increase
pinball radius if it is a true geometric gap/penetration. Decrease
pinball if it is a false one.
****************************************
*** NOTE *** CP = 17.156 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 102 and contact element type 101 has been set up. The
companion pair has real constant set ID 101. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.17100E+17
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.22185E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.32086E-02
Average contact pair depth 0.22185E-02
Average target surface length 0.30136E-02
Default pinball region factor PINB 0.25000
*WARNING*: The default pinball radius may be too small to capture
contacting zone under small sliding assumption. Redefine the pinball
radius if necessary.
The resulting pinball region 0.55462E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.156 TIME= 09:30:01
Min. Initial gap 4.910400625E-04 was detected between contact element
27244 and target element 27038.
Contact is detected due to initial settings.
Max. Geometric gap 5.057185729E-04 has been detected between contact
element 27228 and target element 27033.
*WARNING*: The geometric gap/penetration may be too large. Increase
pinball radius if it is a true geometric gap/penetration. Decrease
pinball if it is a false one.
****************************************
*** NOTE *** CP = 17.156 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 103 and contact element type 103 has been set up. The
companion pair has real constant set ID 104. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.98248E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.40713E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.50184E-02
Average contact pair depth 0.40713E-02
Average target surface length 0.28014E-02
Default pinball region factor PINB 0.25000
*WARNING*: The default pinball radius may be too small to capture
contacting zone under small sliding assumption. Redefine the pinball
radius if necessary.
The resulting pinball region 0.10178E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.156 TIME= 09:30:01
Min. Initial gap 4.9577117E-04 was detected between contact element
27407 and target element 27486.
Contact is detected due to initial settings.
Max. Geometric gap 6.25816756E-04 has been detected between contact
element 27408 and target element 27483.
*WARNING*: The geometric gap/penetration may be too large. Increase
pinball radius if it is a true geometric gap/penetration. Decrease
pinball if it is a false one.
****************************************
*** NOTE *** CP = 17.156 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 104 and contact element type 103 has been set up. The
companion pair has real constant set ID 103. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.98248E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.20033E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.29087E-02
Average contact pair depth 0.20033E-02
Average target surface length 0.48437E-02
Default pinball region factor PINB 0.25000
*WARNING*: The default pinball radius may be too small to capture
contacting zone under small sliding assumption. Redefine the pinball
radius if necessary.
The resulting pinball region 0.50083E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.156 TIME= 09:30:01
Min. Initial gap 4.914419015E-04 was detected between contact element
27445 and target element 27362.
Contact is detected due to initial settings.
Max. Geometric gap 5.00806719E-04 has been detected between contact
element 27432 and target element 27364.
*WARNING*: The geometric gap/penetration may be too large. Increase
pinball radius if it is a true geometric gap/penetration. Decrease
pinball if it is a false one.
****************************************
*** NOTE *** CP = 17.156 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 105 and contact element type 105 has been set up. The
companion pair has real constant set ID 106. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.33684E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.23981E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.67716E-02
Average contact pair depth 0.23981E-02
Average target surface length 0.19342E-01
Default pinball region factor PINB 0.25000
The resulting pinball region 0.59952E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.156 TIME= 09:30:01
Max. Initial penetration 2.775557562E-17 was detected between contact
element 27516 and target element 27560.
****************************************
*** NOTE *** CP = 17.156 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 106 and contact element type 105 has been set up. The
companion pair has real constant set ID 105. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.33684E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.11875E-02
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.20130E-01
Average contact pair depth 0.11875E-01
Average target surface length 0.67254E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.29687E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.156 TIME= 09:30:01
Max. Initial penetration 0 was detected between contact element 27532
and target element 27502.
****************************************
*** NOTE *** CP = 17.156 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 107 and contact element type 107 has been set up. The
companion pair has real constant set ID 108. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.31392E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.24176E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.67716E-02
Average contact pair depth 0.24176E-02
Average target surface length 0.17168E-01
Default pinball region factor PINB 0.25000
The resulting pinball region 0.60439E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.156 TIME= 09:30:01
Max. Initial penetration 2.775557562E-17 was detected between contact
element 27584 and target element 27626.
****************************************
*** NOTE *** CP = 17.156 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 108 and contact element type 107 has been set up. The
companion pair has real constant set ID 107. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.31392E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.12742E-02
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.17767E-01
Average contact pair depth 0.12742E-01
Average target surface length 0.67254E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.31855E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.156 TIME= 09:30:01
Max. Initial penetration 0 was detected between contact element 27598
and target element 27578.
****************************************
*** NOTE *** CP = 17.156 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 109 and contact element type 109 has been set up. The
companion pair has real constant set ID 110. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.61780E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.64746E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.55245E-02
Average contact pair depth 0.64746E-02
Average target surface length 0.37019E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.16187E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.156 TIME= 09:30:01
Min. Initial gap 4.991801484E-04 was detected between contact element
27675 and target element 27707.
Contact is detected due to initial settings.
Max. Geometric gap 6.032847174E-04 has been detected between contact
element 27678 and target element 27705.
****************************************
*** NOTE *** CP = 17.156 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 110 and contact element type 109 has been set up. The
companion pair has real constant set ID 109. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.61780E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.24192E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.35975E-02
Average contact pair depth 0.24192E-02
Average target surface length 0.51776E-02
Default pinball region factor PINB 0.25000
*WARNING*: The default pinball radius may be too small to capture
contacting zone under small sliding assumption. Redefine the pinball
radius if necessary.
The resulting pinball region 0.60481E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.156 TIME= 09:30:01
Min. Initial gap 4.99166501E-04 was detected between contact element
27690 and target element 27644.
Contact is detected due to initial settings.
Max. Geometric gap 6.001E-04 has been detected between contact element
27689 and target element 27639.
*WARNING*: The geometric gap/penetration may be too large. Increase
pinball radius if it is a true geometric gap/penetration. Decrease
pinball if it is a false one.
****************************************
*** NOTE *** CP = 17.156 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 111 and contact element type 111 has been set up. The
companion pair has real constant set ID 112. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.69627E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.57449E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.67038E-02
Average contact pair depth 0.57449E-02
Average target surface length 0.29186E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.14362E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.156 TIME= 09:30:01
Min. Initial gap 4.991010041E-04 was detected between contact element
27725 and target element 27758.
Contact is detected due to initial settings.
Max. Geometric gap 6.608405872E-04 has been detected between contact
element 27739 and target element 27761.
****************************************
*** NOTE *** CP = 17.156 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 112 and contact element type 111 has been set up. The
companion pair has real constant set ID 111. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.69627E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.18117E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.29027E-02
Average contact pair depth 0.18117E-02
Average target surface length 0.64033E-02
Default pinball region factor PINB 0.25000
*WARNING*: The default pinball radius may be too small to capture
contacting zone under small sliding assumption. Redefine the pinball
radius if necessary.
The resulting pinball region 0.45292E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.156 TIME= 09:30:01
No contact was detected for this contact pair.
****************************************
*** NOTE *** CP = 17.156 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 113 and contact element type 113 has been set up. The
companion pair has real constant set ID 114. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.61490E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.65051E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.85897E-02
Average contact pair depth 0.65051E-02
Average target surface length 0.91866E-02
Default pinball region factor PINB 0.25000
*WARNING*: The default pinball radius may be too small to capture
contacting zone under small sliding assumption. Redefine the pinball
radius if necessary.
The resulting pinball region 0.16263E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.156 TIME= 09:30:01
Min. Initial gap 4.880394923E-04 was detected between contact element
27801 and target element 27832.
Contact is detected due to initial settings.
Max. Geometric gap 1.434448592E-03 has been detected between contact
element 27805 and target element 27836.
*WARNING*: The geometric gap/penetration may be too large. Increase
pinball radius if it is a true geometric gap/penetration. Decrease
pinball if it is a false one.
****************************************
*** NOTE *** CP = 17.156 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 114 and contact element type 113 has been set up. The
companion pair has real constant set ID 113. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.61490E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.60082E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.94798E-02
Average contact pair depth 0.60082E-02
Average target surface length 0.81950E-02
Default pinball region factor PINB 0.25000
*WARNING*: The default pinball radius may be too small to capture
contacting zone under small sliding assumption. Redefine the pinball
radius if necessary.
The resulting pinball region 0.15020E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.156 TIME= 09:30:01
Min. Initial gap 4.731531561E-04 was detected between contact element
27820 and target element 27784.
Contact is detected due to initial settings.
Max. Geometric gap 1.388433726E-03 has been detected between contact
element 27816 and target element 27781.
*WARNING*: The geometric gap/penetration may be too large. Increase
pinball radius if it is a true geometric gap/penetration. Decrease
pinball if it is a false one.
****************************************
*** NOTE *** CP = 17.156 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 115 and contact element type 115 has been set up. The
companion pair has real constant set ID 116. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.47825E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.83639E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.10096E-01
Average contact pair depth 0.83639E-02
Average target surface length 0.88240E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.20910E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.156 TIME= 09:30:01
Min. Initial gap 4.765227832E-04 was detected between contact element
27873 and target element 27905.
Contact is detected due to initial settings.
Max. Geometric gap 5.425881594E-04 has been detected between contact
element 27877 and target element 27898.
****************************************
*** NOTE *** CP = 17.156 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 116 and contact element type 115 has been set up. The
companion pair has real constant set ID 115. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.47825E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.60268E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.91068E-02
Average contact pair depth 0.60268E-02
Average target surface length 0.95876E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.15067E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.156 TIME= 09:30:01
Min. Initial gap 4.628556431E-04 was detected between contact element
27892 and target element 27855.
Contact is detected due to initial settings.
Max. Geometric gap 5.876189201E-04 has been detected between contact
element 27883 and target element 27844.
****************************************
*** NOTE *** CP = 17.156 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 117 and contact element type 117 has been set up. The
companion pair has real constant set ID 118. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.99659E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.40137E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.64333E-02
Average contact pair depth 0.40137E-02
Average target surface length 0.35009E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.10034E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.156 TIME= 09:30:01
Min. Initial gap 4.990860243E-04 was detected between contact element
27953 and target element 27996.
Contact is detected due to initial settings.
Max. Geometric gap 6.054222446E-04 has been detected between contact
element 27959 and target element 27998.
*WARNING*: The geometric gap/penetration may be too large. Increase
pinball radius if it is a true geometric gap/penetration. Decrease
pinball if it is a false one.
****************************************
*** NOTE *** CP = 17.156 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 118 and contact element type 117 has been set up. The
companion pair has real constant set ID 117. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.99659E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.19156E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.34841E-02
Average contact pair depth 0.19156E-02
Average target surface length 0.59569E-02
Default pinball region factor PINB 0.25000
*WARNING*: The default pinball radius may be too small to capture
contacting zone under small sliding assumption. Redefine the pinball
radius if necessary.
The resulting pinball region 0.47891E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.156 TIME= 09:30:01
No contact was detected for this contact pair.
****************************************
*** NOTE *** CP = 17.156 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 119 and contact element type 119 has been set up. The
companion pair has real constant set ID 120. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.59135E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.67641E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.11896E-01
Average contact pair depth 0.67641E-02
Average target surface length 0.91805E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.16910E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.156 TIME= 09:30:01
Min. Initial gap 4.616567429E-04 was detected between contact element
28027 and target element 28062.
Contact is detected due to initial settings.
Max. Geometric gap 5.329327707E-04 has been detected between contact
element 28024 and target element 28058.
****************************************
*** NOTE *** CP = 17.156 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 120 and contact element type 119 has been set up. The
companion pair has real constant set ID 119. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.59135E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.59744E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.94747E-02
Average contact pair depth 0.59744E-02
Average target surface length 0.11153E-01
Default pinball region factor PINB 0.25000
The resulting pinball region 0.14936E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.156 TIME= 09:30:01
Min. Initial gap 4.459523403E-04 was detected between contact element
28050 and target element 28007.
Contact is detected due to initial settings.
Max. Geometric gap 5.450774883E-04 has been detected between contact
element 28046 and target element 28004.
****************************************
*** NOTE *** CP = 17.156 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 121 and contact element type 121 has been set up. The
companion pair has real constant set ID 122. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.49021E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.65732E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.10900E-01
Average contact pair depth 0.65732E-02
Average target surface length 0.91805E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.16433E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.156 TIME= 09:30:01
Min. Initial gap 4.598042426E-04 was detected between contact element
28100 and target element 28129.
Contact is detected due to initial settings.
Max. Geometric gap 4.987714614E-04 has been detected between contact
element 28102 and target element 28118.
****************************************
*** NOTE *** CP = 17.156 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 122 and contact element type 121 has been set up. The
companion pair has real constant set ID 121. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.49021E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.81597E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.94747E-02
Average contact pair depth 0.81597E-02
Average target surface length 0.10212E-01
Default pinball region factor PINB 0.25000
The resulting pinball region 0.20399E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.156 TIME= 09:30:01
Min. Initial gap 4.494279709E-04 was detected between contact element
28117 and target element 28079.
Contact is detected due to initial settings.
Max. Geometric gap 5.946212391E-04 has been detected between contact
element 28114 and target element 28070.
****************************************
*** NOTE *** CP = 17.156 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 123 and contact element type 123 has been set up. The
companion pair has real constant set ID 124. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.52305E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.22691E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.36454E-02
Average contact pair depth 0.22691E-02
Average target surface length 0.12050E-01
Default pinball region factor PINB 0.25000
The resulting pinball region 0.56728E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.156 TIME= 09:30:01
Max. Initial penetration 6.938893904E-18 was detected between contact
element 28143 and target element 28170.
****************************************
*** NOTE *** CP = 17.156 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 124 and contact element type 123 has been set up. The
companion pair has real constant set ID 123. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.52305E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.76475E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.12431E-01
Average contact pair depth 0.76475E-02
Average target surface length 0.37691E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.19119E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.156 TIME= 09:30:01
Max. Initial penetration 6.938893904E-18 was detected between contact
element 28159 and target element 28134.
****************************************
*** NOTE *** CP = 17.156 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 125 and contact element type 125 has been set up. The
companion pair has real constant set ID 126. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.66769E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.24261E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.36454E-02
Average contact pair depth 0.24261E-02
Average target surface length 0.11869E-01
Default pinball region factor PINB 0.25000
The resulting pinball region 0.60652E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.156 TIME= 09:30:01
Max. Initial penetration 6.938893904E-18 was detected between contact
element 28199 and target element 28219.
****************************************
*** NOTE *** CP = 17.156 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 126 and contact element type 125 has been set up. The
companion pair has real constant set ID 125. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.66769E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.59908E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.12246E-01
Average contact pair depth 0.59908E-02
Average target surface length 0.37691E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.14977E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.156 TIME= 09:30:01
Max. Initial penetration 6.938893904E-18 was detected between contact
element 28205 and target element 28186.
****************************************
*** NOTE *** CP = 17.156 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 127 and contact element type 127 has been set up. The
companion pair has real constant set ID 128. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.48217E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.22750E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.36454E-02
Average contact pair depth 0.22750E-02
Average target surface length 0.10279E-01
Default pinball region factor PINB 0.25000
The resulting pinball region 0.56876E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.156 TIME= 09:30:01
Max. Initial penetration 1.736955052E-04 was detected between contact
element 28253 and target element 28272.
You may move entire target surface by : x= -8.197647376E-20, y=
-1.736955052E-04, z= -5.340808408E-20,to reduce initial penetration.
****************************************
*** NOTE *** CP = 17.156 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 128 and contact element type 127 has been set up. The
companion pair has real constant set ID 127. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.48217E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.82959E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.10684E-01
Average contact pair depth 0.82959E-02
Average target surface length 0.37691E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.20740E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.156 TIME= 09:30:01
Max. Initial penetration 6.195620121E-05 was detected between contact
element 28255 and target element 28241.
You may move entire target surface by : x= 8.98413284E-06, y=
6.129957E-05, z= 4.679138581E-07,to reduce initial penetration.
****************************************
*** NOTE *** CP = 17.156 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 129 and contact element type 129 has been set up. The
companion pair has real constant set ID 130. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.59273E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.24261E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.36454E-02
Average contact pair depth 0.24261E-02
Average target surface length 0.12056E-01
Default pinball region factor PINB 0.25000
The resulting pinball region 0.60652E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.156 TIME= 09:30:01
Max. Initial penetration 2.775557562E-17 was detected between contact
element 28305 and target element 28329.
****************************************
*** NOTE *** CP = 17.156 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 130 and contact element type 129 has been set up. The
companion pair has real constant set ID 129. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.59273E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.67485E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.12438E-01
Average contact pair depth 0.67485E-02
Average target surface length 0.37691E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.16871E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.156 TIME= 09:30:01
Max. Initial penetration 5.551115123E-17 was detected between contact
element 28315 and target element 28293.
****************************************
*** NOTE *** CP = 17.156 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 131 and contact element type 131 has been set up. The
companion pair has real constant set ID 132. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.16371E+17
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.24434E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.30579E-02
Average contact pair depth 0.24434E-02
Average target surface length 0.30914E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.61085E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.156 TIME= 09:30:01
Min. Initial gap 4.879144707E-04 was detected between contact element
28485 and target element 28688.
Contact is detected due to initial settings.
Max. Geometric gap 6.001E-04 has been detected between contact element
28514 and target element 28698.
*WARNING*: The geometric gap/penetration may be too large. Increase
pinball radius if it is a true geometric gap/penetration. Decrease
pinball if it is a false one.
****************************************
*** NOTE *** CP = 17.156 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 132 and contact element type 131 has been set up. The
companion pair has real constant set ID 131. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.16371E+17
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.22155E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.32181E-02
Average contact pair depth 0.22155E-02
Average target surface length 0.29474E-02
Default pinball region factor PINB 0.25000
*WARNING*: The default pinball radius may be too small to capture
contacting zone under small sliding assumption. Redefine the pinball
radius if necessary.
The resulting pinball region 0.55386E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.156 TIME= 09:30:01
Min. Initial gap 4.913508331E-04 was detected between contact element
28594 and target element 28375.
Contact is detected due to initial settings.
Max. Geometric gap 5.057078782E-04 has been detected between contact
element 28577 and target element 28376.
*WARNING*: The geometric gap/penetration may be too large. Increase
pinball radius if it is a true geometric gap/penetration. Decrease
pinball if it is a false one.
****************************************
*** NOTE *** CP = 17.156 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 133 and contact element type 133 has been set up. The
companion pair has real constant set ID 134. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.93276E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.42884E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.45003E-02
Average contact pair depth 0.42884E-02
Average target surface length 0.28005E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.10721E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.156 TIME= 09:30:01
Min. Initial gap 4.890477239E-04 was detected between contact element
28820 and target element 28896.
Contact is detected due to initial settings.
Max. Geometric gap 5.056590656E-04 has been detected between contact
element 28812 and target element 28891.
****************************************
*** NOTE *** CP = 17.156 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 134 and contact element type 133 has been set up. The
companion pair has real constant set ID 133. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.93276E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.21278E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.28894E-02
Average contact pair depth 0.21278E-02
Average target surface length 0.43589E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.53196E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.156 TIME= 09:30:01
Min. Initial gap 4.900971609E-04 was detected between contact element
28862 and target element 28755.
Contact is detected due to initial settings.
Max. Geometric gap 5.07768796E-04 has been detected between contact
element 28856 and target element 28765.
*WARNING*: The geometric gap/penetration may be too large. Increase
pinball radius if it is a true geometric gap/penetration. Decrease
pinball if it is a false one.
****************************************
*** NOTE *** CP = 17.156 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 135 and contact element type 135 has been set up. The
companion pair has real constant set ID 136. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.52236E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.47792E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.58138E-02
Average contact pair depth 0.47792E-02
Average target surface length 0.14537E-01
Default pinball region factor PINB 0.25000
The resulting pinball region 0.11948E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.156 TIME= 09:30:01
Min. Initial gap 4.666536896E-04 was detected between contact element
28998 and target element 29066.
Contact is detected due to initial settings.
Max. Geometric gap 1.044318799E-03 has been detected between contact
element 29029 and target element 29067.
*WARNING*: The geometric gap/penetration may be too large. Increase
pinball radius if it is a true geometric gap/penetration. Decrease
pinball if it is a false one.
****************************************
*** NOTE *** CP = 17.156 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 136 and contact element type 135 has been set up. The
companion pair has real constant set ID 135. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.52236E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.76575E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.15620E-01
Average contact pair depth 0.76575E-02
Average target surface length 0.56598E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.19144E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.156 TIME= 09:30:01
Min. Initial gap 4.662224708E-04 was detected between contact element
29055 and target element 28948.
Contact is detected due to initial settings.
Max. Geometric gap 1.009698691E-03 has been detected between contact
element 29058 and target element 28957.
*WARNING*: The geometric gap/penetration may be too large. Increase
pinball radius if it is a true geometric gap/penetration. Decrease
pinball if it is a false one.
****************************************
*** NOTE *** CP = 17.156 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 137 and contact element type 137 has been set up. The
companion pair has real constant set ID 138. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.47172E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.36190E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.48409E-02
Average contact pair depth 0.36190E-02
Average target surface length 0.14537E-01
Default pinball region factor PINB 0.25000
The resulting pinball region 0.90475E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.156 TIME= 09:30:01
Min. Initial gap 4.661935734E-04 was detected between contact element
29232 and target element 29293.
Contact is detected due to initial settings.
Max. Geometric gap 4.917324487E-04 has been detected between contact
element 29229 and target element 29288.
*WARNING*: The geometric gap/penetration may be too large. Increase
pinball radius if it is a true geometric gap/penetration. Decrease
pinball if it is a false one.
****************************************
*** NOTE *** CP = 17.156 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 138 and contact element type 137 has been set up. The
companion pair has real constant set ID 137. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.47172E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.84797E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.15620E-01
Average contact pair depth 0.84797E-02
Average target surface length 0.47202E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.21199E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.156 TIME= 09:30:01
Min. Initial gap 4.662654477E-04 was detected between contact element
29272 and target element 29132.
Contact is detected due to initial settings.
Max. Geometric gap 4.863963432E-04 has been detected between contact
element 29277 and target element 29143.
****************************************
*** NOTE *** CP = 17.156 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 139 and contact element type 139 has been set up. The
companion pair has real constant set ID 140. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.55911E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.24430E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.67716E-02
Average contact pair depth 0.24430E-02
Average target surface length 0.13775E-01
Default pinball region factor PINB 0.25000
The resulting pinball region 0.61076E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.156 TIME= 09:30:01
Max. Initial penetration 3.469446952E-18 was detected between contact
element 29317 and target element 29358.
****************************************
*** NOTE *** CP = 17.156 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 140 and contact element type 139 has been set up. The
companion pair has real constant set ID 139. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.55911E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.71543E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.14111E-01
Average contact pair depth 0.71543E-02
Average target surface length 0.67254E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.17886E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.156 TIME= 09:30:01
Max. Initial penetration 3.469446952E-18 was detected between contact
element 29329 and target element 29309.
****************************************
*** NOTE *** CP = 17.156 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 141 and contact element type 141 has been set up. The
companion pair has real constant set ID 142. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.34840E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.23792E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.67716E-02
Average contact pair depth 0.23792E-02
Average target surface length 0.12803E-01
Default pinball region factor PINB 0.25000
The resulting pinball region 0.59479E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.156 TIME= 09:30:01
Max. Initial penetration 1.106700545E-04 was detected between contact
element 29385 and target element 29419.
You may move entire target surface by : x= 1.698393613E-20, y=
-1.106700545E-04, z= 1.940768735E-21,to reduce initial penetration.
****************************************
*** NOTE *** CP = 17.156 TIME= 09:30:01
Symmetric Deformable- deformable contact pair identified by real
constant set 142 and contact element type 141 has been set up. The
companion pair has real constant set ID 141. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.34840E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.11481E-02
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.13407E-01
Average contact pair depth 0.11481E-01
Average target surface length 0.67254E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.28702E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.156 TIME= 09:30:01
Max. Initial penetration 1.113237698E-04 was detected between contact
element 29394 and target element 29368.
You may move entire target surface by : x= 6.753189682E-07, y=
1.0932821E-04, z= 2.097303449E-05,to reduce initial penetration.
****************************************
*** WARNING *** CP = 17.156 TIME= 09:30:01
Maximum contact stiffness 1.759623794E+16 is too big which can cause an
accuracy problem, you may scale the force units in the model.
D I S T R I B U T E D D O M A I N D E C O M P O S E R
...Number of elements: 20256
...Number of nodes: 30685
...Decompose to 2 CPU domains
...Element load balance ratio = 1.042
L O A D S T E P O P T I O N S
LOAD STEP NUMBER. . . . . . . . . . . . . . . . 1
TIME AT END OF THE LOAD STEP. . . . . . . . . . 1.0000
NUMBER OF SUBSTEPS. . . . . . . . . . . . . . . 1
STEP CHANGE BOUNDARY CONDITIONS . . . . . . . . NO
PRINT OUTPUT CONTROLS . . . . . . . . . . . . .NO PRINTOUT
DATABASE OUTPUT CONTROLS
ITEM FREQUENCY COMPONENT
ALL NONE
NSOL ALL
RSOL ALL
EANG ALL
ETMP ALL
VENG ALL
STRS ALL
EPEL ALL
EPPL ALL
EPTH ALL
CONT ALL
SOLUTION MONITORING INFO IS WRITTEN TO FILE= file.mntr
*** NOTE *** CP = 17.594 TIME= 09:30:02
Symmetric Deformable- deformable contact pair identified by real
constant set 55 and contact element type 55 has been set up. The
companion pair has real constant set ID 56. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.17596E+17
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.22732E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.31042E-02
Average contact pair depth 0.22732E-02
Average target surface length 0.30935E-02
Default pinball region factor PINB 0.25000
*WARNING*: The default pinball radius may be too small to capture
contacting zone under small sliding assumption. Redefine the pinball
radius if necessary.
The resulting pinball region 0.56830E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.594 TIME= 09:30:02
Min. Initial gap 4.892041643E-04 was detected between contact element
24597 and target element 24797.
Contact is detected due to initial settings.
Max. Geometric gap 5.108346823E-04 has been detected between contact
element 24608 and target element 24805.
*WARNING*: The geometric gap/penetration may be too large. Increase
pinball radius if it is a true geometric gap/penetration. Decrease
pinball if it is a false one.
****************************************
*** NOTE *** CP = 17.594 TIME= 09:30:02
Symmetric Deformable- deformable contact pair identified by real
constant set 56 and contact element type 55 has been set up. The
companion pair has real constant set ID 55. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.17596E+17
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.22155E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.32205E-02
Average contact pair depth 0.22155E-02
Average target surface length 0.29897E-02
Default pinball region factor PINB 0.25000
*WARNING*: The default pinball radius may be too small to capture
contacting zone under small sliding assumption. Redefine the pinball
radius if necessary.
The resulting pinball region 0.55388E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.594 TIME= 09:30:02
Min. Initial gap 4.914598591E-04 was detected between contact element
24703 and target element 24481.
Contact is detected due to initial settings.
Max. Geometric gap 5.103830541E-04 has been detected between contact
element 24711 and target element 24504.
*WARNING*: The geometric gap/penetration may be too large. Increase
pinball radius if it is a true geometric gap/penetration. Decrease
pinball if it is a false one.
****************************************
*** NOTE *** CP = 17.594 TIME= 09:30:02
Symmetric Deformable- deformable contact pair identified by real
constant set 57 and contact element type 57 has been set up. The
companion pair has real constant set ID 58. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.10201E+17
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.39212E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.46042E-02
Average contact pair depth 0.39212E-02
Average target surface length 0.28864E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.98030E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.594 TIME= 09:30:02
Min. Initial gap 4.910065956E-04 was detected between contact element
24874 and target element 24952.
Contact is detected due to initial settings.
Max. Geometric gap 5.061191669E-04 has been detected between contact
element 24876 and target element 24950.
*WARNING*: The geometric gap/penetration may be too large. Increase
pinball radius if it is a true geometric gap/penetration. Decrease
pinball if it is a false one.
****************************************
*** NOTE *** CP = 17.594 TIME= 09:30:02
Symmetric Deformable- deformable contact pair identified by real
constant set 58 and contact element type 57 has been set up. The
companion pair has real constant set ID 57. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.10201E+17
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.25334E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.29829E-02
Average contact pair depth 0.25334E-02
Average target surface length 0.44708E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.63336E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.594 TIME= 09:30:02
Min. Initial gap 4.920431168E-04 was detected between contact element
24917 and target element 24833.
Contact is detected due to initial settings.
Max. Geometric gap 5.061138968E-04 has been detected between contact
element 24909 and target element 24836.
*WARNING*: The geometric gap/penetration may be too large. Increase
pinball radius if it is a true geometric gap/penetration. Decrease
pinball if it is a false one.
****************************************
*** NOTE *** CP = 17.594 TIME= 09:30:02
Symmetric Deformable- deformable contact pair identified by real
constant set 61 and contact element type 61 has been set up. The
companion pair has real constant set ID 62. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.51152E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.33229E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.49022E-02
Average contact pair depth 0.33229E-02
Average target surface length 0.14531E-01
Default pinball region factor PINB 0.25000
*WARNING*: The default pinball radius may be too small to capture
contacting zone under small sliding assumption. Redefine the pinball
radius if necessary.
The resulting pinball region 0.83073E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.594 TIME= 09:30:02
Min. Initial gap 2.598040708E-04 was detected between contact element
25254 and target element 25302.
Contact is detected due to initial settings.
Max. Geometric gap 6.188006116E-04 has been detected between contact
element 25275 and target element 25298.
*WARNING*: The geometric gap/penetration may be too large. Increase
pinball radius if it is a true geometric gap/penetration. Decrease
pinball if it is a false one.
****************************************
*** NOTE *** CP = 17.594 TIME= 09:30:02
Symmetric Deformable- deformable contact pair identified by real
constant set 62 and contact element type 61 has been set up. The
companion pair has real constant set ID 61. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.51152E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.78199E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.15617E-01
Average contact pair depth 0.78199E-02
Average target surface length 0.47793E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.19550E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.594 TIME= 09:30:02
Min. Initial gap 2.739881357E-04 was detected between contact element
25290 and target element 25174.
Contact is detected due to initial settings.
Max. Geometric gap 5.011163479E-04 has been detected between contact
element 25283 and target element 25162.
****************************************
*** NOTE *** CP = 17.594 TIME= 09:30:02
Symmetric Deformable- deformable contact pair identified by real
constant set 65 and contact element type 65 has been set up. The
companion pair has real constant set ID 66. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.47822E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.22750E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.36454E-02
Average contact pair depth 0.22750E-02
Average target surface length 0.98585E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.56876E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.594 TIME= 09:30:02
Max. Initial penetration 1.736955052E-04 was detected between contact
element 25361 and target element 25388.
You may move entire target surface by : x= 2.91539766E-20, y=
-1.736955052E-04, z= -3.343422002E-20,to reduce initial penetration.
****************************************
*** NOTE *** CP = 17.594 TIME= 09:30:02
Symmetric Deformable- deformable contact pair identified by real
constant set 66 and contact element type 65 has been set up. The
companion pair has real constant set ID 65. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.47822E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.83643E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.10201E-01
Average contact pair depth 0.83643E-02
Average target surface length 0.37691E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.20911E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.594 TIME= 09:30:02
Max. Initial penetration 6.195620121E-05 was detected between contact
element 25372 and target element 25349.
You may move entire target surface by : x= -8.98413284E-06, y=
6.129957E-05, z= 4.679138581E-07,to reduce initial penetration.
****************************************
*** NOTE *** CP = 17.594 TIME= 09:30:02
Symmetric Deformable- deformable contact pair identified by real
constant set 69 and contact element type 69 has been set up. The
companion pair has real constant set ID 70. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.37743E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.24103E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.67716E-02
Average contact pair depth 0.24103E-02
Average target surface length 0.12921E-01
Default pinball region factor PINB 0.25000
The resulting pinball region 0.60258E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.594 TIME= 09:30:02
Max. Initial penetration 1.021405789E-04 was detected between contact
element 25492 and target element 25546.
You may move entire target surface by : x= 3.178767662E-19, y=
1.021405789E-04, z= -4.486295031E-19,to reduce initial penetration.
****************************************
*** NOTE *** CP = 17.594 TIME= 09:30:02
Symmetric Deformable- deformable contact pair identified by real
constant set 70 and contact element type 69 has been set up. The
companion pair has real constant set ID 69. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.37743E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.10598E-02
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.13550E-01
Average contact pair depth 0.10598E-01
Average target surface length 0.67254E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.26495E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.594 TIME= 09:30:02
Max. Initial penetration 7.29363013E-05 was detected between contact
element 25520 and target element 25475.
You may move entire target surface by : x= -4.791479912E-07, y=
-7.201771111E-05, z= 1.152925631E-05,to reduce initial penetration.
****************************************
*** NOTE *** CP = 17.594 TIME= 09:30:02
Symmetric Deformable- deformable contact pair identified by real
constant set 73 and contact element type 73 has been set up. The
companion pair has real constant set ID 74. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.48536E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.21852E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.36454E-02
Average contact pair depth 0.21852E-02
Average target surface length 0.96458E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.54630E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.594 TIME= 09:30:02
Max. Initial penetration 1.736954878E-04 was detected between contact
element 25627 and target element 25656.
You may move entire target surface by : x= -1.162760459E-18, y=
1.736954878E-04, z= 1.256153359E-18,to reduce initial penetration.
****************************************
*** NOTE *** CP = 17.594 TIME= 09:30:02
Symmetric Deformable- deformable contact pair identified by real
constant set 74 and contact element type 73 has been set up. The
companion pair has real constant set ID 73. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.48536E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.82412E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.99777E-02
Average contact pair depth 0.82412E-02
Average target surface length 0.37691E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.20603E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.594 TIME= 09:30:02
Max. Initial penetration 6.195620923E-05 was detected between contact
element 25639 and target element 25615.
You may move entire target surface by : x= -8.984135126E-06, y=
-6.129957778E-05, z= 4.679135334E-07,to reduce initial penetration.
****************************************
*** NOTE *** CP = 17.594 TIME= 09:30:02
Symmetric Deformable- deformable contact pair identified by real
constant set 75 and contact element type 75 has been set up. The
companion pair has real constant set ID 76. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.10596E+17
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.37750E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.57784E-02
Average contact pair depth 0.37750E-02
Average target surface length 0.35009E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.94375E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.594 TIME= 09:30:02
Min. Initial gap 4.990860284E-04 was detected between contact element
25711 and target element 25754.
Contact is detected due to initial settings.
Max. Geometric gap 6.638924593E-04 has been detected between contact
element 25716 and target element 25755.
*WARNING*: The geometric gap/penetration may be too large. Increase
pinball radius if it is a true geometric gap/penetration. Decrease
pinball if it is a false one.
****************************************
*** NOTE *** CP = 17.594 TIME= 09:30:02
Symmetric Deformable- deformable contact pair identified by real
constant set 76 and contact element type 75 has been set up. The
companion pair has real constant set ID 75. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.10596E+17
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.19156E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.34841E-02
Average contact pair depth 0.19156E-02
Average target surface length 0.53755E-02
Default pinball region factor PINB 0.25000
*WARNING*: The default pinball radius may be too small to capture
contacting zone under small sliding assumption. Redefine the pinball
radius if necessary.
The resulting pinball region 0.47891E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.594 TIME= 09:30:02
No contact was detected for this contact pair.
****************************************
*** NOTE *** CP = 17.594 TIME= 09:30:02
Symmetric Deformable- deformable contact pair identified by real
constant set 81 and contact element type 81 has been set up. The
companion pair has real constant set ID 82. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.60222E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.57913E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.98935E-02
Average contact pair depth 0.57913E-02
Average target surface length 0.86839E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.14478E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.594 TIME= 09:30:02
Min. Initial gap 4.441485883E-04 was detected between contact element
25912 and target element 25935.
Contact is detected due to initial settings.
Max. Geometric gap 5.632278433E-04 has been detected between contact
element 25913 and target element 25938.
****************************************
*** NOTE *** CP = 17.594 TIME= 09:30:02
Symmetric Deformable- deformable contact pair identified by real
constant set 82 and contact element type 81 has been set up. The
companion pair has real constant set ID 81. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.60222E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.66421E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.88969E-02
Average contact pair depth 0.66421E-02
Average target surface length 0.92954E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.16605E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.594 TIME= 09:30:02
Min. Initial gap 4.294073687E-04 was detected between contact element
25923 and target element 25890.
Contact is detected due to initial settings.
Max. Geometric gap 6.261542594E-04 has been detected between contact
element 25925 and target element 25884.
****************************************
*** NOTE *** CP = 17.594 TIME= 09:30:02
Symmetric Deformable- deformable contact pair identified by real
constant set 83 and contact element type 83 has been set up. The
companion pair has real constant set ID 84. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.60735E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.22691E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.36454E-02
Average contact pair depth 0.22691E-02
Average target surface length 0.11876E-01
Default pinball region factor PINB 0.25000
The resulting pinball region 0.56728E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.594 TIME= 09:30:02
Max. Initial penetration 5.551115123E-17 was detected between contact
element 25963 and target element 25989.
****************************************
*** NOTE *** CP = 17.594 TIME= 09:30:02
Symmetric Deformable- deformable contact pair identified by real
constant set 84 and contact element type 83 has been set up. The
companion pair has real constant set ID 83. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.60735E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.65860E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.12255E-01
Average contact pair depth 0.65860E-02
Average target surface length 0.37691E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.16465E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.594 TIME= 09:30:02
Max. Initial penetration 8.326672685E-17 was detected between contact
element 25972 and target element 25946.
****************************************
*** NOTE *** CP = 17.594 TIME= 09:30:02
Symmetric Deformable- deformable contact pair identified by real
constant set 85 and contact element type 85 has been set up. The
companion pair has real constant set ID 86. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.53384E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.22750E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.36454E-02
Average contact pair depth 0.22750E-02
Average target surface length 0.10238E-01
Default pinball region factor PINB 0.25000
The resulting pinball region 0.56876E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.594 TIME= 09:30:02
Max. Initial penetration 1.736954878E-04 was detected between contact
element 26009 and target element 26050.
You may move entire target surface by : x= 1.054076711E-18, y=
1.736954878E-04, z= 1.298304891E-18,to reduce initial penetration.
****************************************
*** NOTE *** CP = 17.594 TIME= 09:30:02
Symmetric Deformable- deformable contact pair identified by real
constant set 86 and contact element type 85 has been set up. The
companion pair has real constant set ID 85. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.53384E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.74929E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.10632E-01
Average contact pair depth 0.74929E-02
Average target surface length 0.37691E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.18732E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.594 TIME= 09:30:02
Max. Initial penetration 6.195620924E-05 was detected between contact
element 26033 and target element 25997.
You may move entire target surface by : x= 8.984135126E-06, y=
-6.129957778E-05, z= 4.679135334E-07,to reduce initial penetration.
****************************************
*** NOTE *** CP = 17.594 TIME= 09:30:02
Symmetric Deformable- deformable contact pair identified by real
constant set 87 and contact element type 87 has been set up. The
companion pair has real constant set ID 88. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.94595E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.42286E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.57472E-02
Average contact pair depth 0.42286E-02
Average target surface length 0.37019E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.10571E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.594 TIME= 09:30:02
Min. Initial gap 4.990834106E-04 was detected between contact element
26104 and target element 26139.
Contact is detected due to initial settings.
Max. Geometric gap 6.97250943E-04 has been detected between contact
element 26113 and target element 26137.
*WARNING*: The geometric gap/penetration may be too large. Increase
pinball radius if it is a true geometric gap/penetration. Decrease
pinball if it is a false one.
****************************************
*** NOTE *** CP = 17.594 TIME= 09:30:02
Symmetric Deformable- deformable contact pair identified by real
constant set 88 and contact element type 87 has been set up. The
companion pair has real constant set ID 87. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.94595E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.24192E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.35975E-02
Average contact pair depth 0.24192E-02
Average target surface length 0.54068E-02
Default pinball region factor PINB 0.25000
*WARNING*: The default pinball radius may be too small to capture
contacting zone under small sliding assumption. Redefine the pinball
radius if necessary.
The resulting pinball region 0.60481E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.594 TIME= 09:30:02
Min. Initial gap 4.991700363E-04 was detected between contact element
26127 and target element 26073.
Contact is detected due to initial settings.
Max. Geometric gap 6.001E-04 has been detected between contact element
26117 and target element 26061.
*WARNING*: The geometric gap/penetration may be too large. Increase
pinball radius if it is a true geometric gap/penetration. Decrease
pinball if it is a false one.
****************************************
*** NOTE *** CP = 17.594 TIME= 09:30:02
Symmetric Deformable- deformable contact pair identified by real
constant set 93 and contact element type 93 has been set up. The
companion pair has real constant set ID 94. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.17429E+17
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.22950E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.31471E-02
Average contact pair depth 0.22950E-02
Average target surface length 0.30838E-02
Default pinball region factor PINB 0.25000
*WARNING*: The default pinball radius may be too small to capture
contacting zone under small sliding assumption. Redefine the pinball
radius if necessary.
The resulting pinball region 0.57376E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.594 TIME= 09:30:02
Min. Initial gap 4.918864337E-04 was detected between contact element
26465 and target element 26665.
Contact is detected due to initial settings.
Max. Geometric gap 5.046381826E-04 has been detected between contact
element 26460 and target element 26660.
*WARNING*: The geometric gap/penetration may be too large. Increase
pinball radius if it is a true geometric gap/penetration. Decrease
pinball if it is a false one.
****************************************
*** NOTE *** CP = 17.594 TIME= 09:30:02
Symmetric Deformable- deformable contact pair identified by real
constant set 94 and contact element type 93 has been set up. The
companion pair has real constant set ID 93. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.17429E+17
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.22169E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.32086E-02
Average contact pair depth 0.22169E-02
Average target surface length 0.30320E-02
Default pinball region factor PINB 0.25000
*WARNING*: The default pinball radius may be too small to capture
contacting zone under small sliding assumption. Redefine the pinball
radius if necessary.
The resulting pinball region 0.55424E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.594 TIME= 09:30:02
Min. Initial gap 4.910400799E-04 was detected between contact element
26570 and target element 26362.
Contact is detected due to initial settings.
Max. Geometric gap 5.057185782E-04 has been detected between contact
element 26554 and target element 26357.
*WARNING*: The geometric gap/penetration may be too large. Increase
pinball radius if it is a true geometric gap/penetration. Decrease
pinball if it is a false one.
****************************************
*** NOTE *** CP = 17.594 TIME= 09:30:02
Symmetric Deformable- deformable contact pair identified by real
constant set 97 and contact element type 97 has been set up. The
companion pair has real constant set ID 98. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.31133E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.24430E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.67716E-02
Average contact pair depth 0.24430E-02
Average target surface length 0.19342E-01
Default pinball region factor PINB 0.25000
The resulting pinball region 0.61076E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.594 TIME= 09:30:02
Max. Initial penetration 2.775557562E-17 was detected between contact
element 26842 and target element 26873.
****************************************
*** NOTE *** CP = 17.594 TIME= 09:30:02
Symmetric Deformable- deformable contact pair identified by real
constant set 98 and contact element type 97 has been set up. The
companion pair has real constant set ID 97. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.31133E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.12848E-02
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.20130E-01
Average contact pair depth 0.12848E-01
Average target surface length 0.67254E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.32120E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.594 TIME= 09:30:02
Max. Initial penetration 2.775557562E-17 was detected between contact
element 26870 and target element 26834.
****************************************
*** NOTE *** CP = 17.594 TIME= 09:30:02
Symmetric Deformable- deformable contact pair identified by real
constant set 105 and contact element type 105 has been set up. The
companion pair has real constant set ID 106. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.33684E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.23981E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.67716E-02
Average contact pair depth 0.23981E-02
Average target surface length 0.19342E-01
Default pinball region factor PINB 0.25000
The resulting pinball region 0.59952E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.594 TIME= 09:30:02
Max. Initial penetration 2.775557562E-17 was detected between contact
element 27516 and target element 27560.
****************************************
*** NOTE *** CP = 17.594 TIME= 09:30:02
Symmetric Deformable- deformable contact pair identified by real
constant set 106 and contact element type 105 has been set up. The
companion pair has real constant set ID 105. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.33684E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.11875E-02
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.20130E-01
Average contact pair depth 0.11875E-01
Average target surface length 0.67254E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.29687E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.594 TIME= 09:30:02
Max. Initial penetration 0 was detected between contact element 27532
and target element 27502.
****************************************
*** NOTE *** CP = 17.594 TIME= 09:30:02
Symmetric Deformable- deformable contact pair identified by real
constant set 113 and contact element type 113 has been set up. The
companion pair has real constant set ID 114. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.61490E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.65051E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.85897E-02
Average contact pair depth 0.65051E-02
Average target surface length 0.91866E-02
Default pinball region factor PINB 0.25000
*WARNING*: The default pinball radius may be too small to capture
contacting zone under small sliding assumption. Redefine the pinball
radius if necessary.
The resulting pinball region 0.16263E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.594 TIME= 09:30:02
Min. Initial gap 4.880394923E-04 was detected between contact element
27801 and target element 27832.
Contact is detected due to initial settings.
Max. Geometric gap 1.434448592E-03 has been detected between contact
element 27805 and target element 27836.
*WARNING*: The geometric gap/penetration may be too large. Increase
pinball radius if it is a true geometric gap/penetration. Decrease
pinball if it is a false one.
****************************************
*** NOTE *** CP = 17.594 TIME= 09:30:02
Symmetric Deformable- deformable contact pair identified by real
constant set 114 and contact element type 113 has been set up. The
companion pair has real constant set ID 113. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.61490E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.60082E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.94798E-02
Average contact pair depth 0.60082E-02
Average target surface length 0.81950E-02
Default pinball region factor PINB 0.25000
*WARNING*: The default pinball radius may be too small to capture
contacting zone under small sliding assumption. Redefine the pinball
radius if necessary.
The resulting pinball region 0.15020E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.594 TIME= 09:30:02
Min. Initial gap 4.731531561E-04 was detected between contact element
27820 and target element 27784.
Contact is detected due to initial settings.
Max. Geometric gap 1.388433726E-03 has been detected between contact
element 27816 and target element 27781.
*WARNING*: The geometric gap/penetration may be too large. Increase
pinball radius if it is a true geometric gap/penetration. Decrease
pinball if it is a false one.
****************************************
*** NOTE *** CP = 17.594 TIME= 09:30:02
Symmetric Deformable- deformable contact pair identified by real
constant set 115 and contact element type 115 has been set up. The
companion pair has real constant set ID 116. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.47825E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.83639E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.10096E-01
Average contact pair depth 0.83639E-02
Average target surface length 0.88240E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.20910E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.594 TIME= 09:30:02
Min. Initial gap 4.765227832E-04 was detected between contact element
27873 and target element 27905.
Contact is detected due to initial settings.
Max. Geometric gap 5.425881594E-04 has been detected between contact
element 27877 and target element 27898.
****************************************
*** NOTE *** CP = 17.594 TIME= 09:30:02
Symmetric Deformable- deformable contact pair identified by real
constant set 116 and contact element type 115 has been set up. The
companion pair has real constant set ID 115. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.47825E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.60268E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.91068E-02
Average contact pair depth 0.60268E-02
Average target surface length 0.95876E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.15067E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.594 TIME= 09:30:02
Min. Initial gap 4.628556431E-04 was detected between contact element
27892 and target element 27855.
Contact is detected due to initial settings.
Max. Geometric gap 5.876189201E-04 has been detected between contact
element 27883 and target element 27844.
****************************************
*** NOTE *** CP = 17.594 TIME= 09:30:02
Symmetric Deformable- deformable contact pair identified by real
constant set 117 and contact element type 117 has been set up. The
companion pair has real constant set ID 118. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.99659E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.40137E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.64333E-02
Average contact pair depth 0.40137E-02
Average target surface length 0.35009E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.10034E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.594 TIME= 09:30:02
Min. Initial gap 4.990860243E-04 was detected between contact element
27953 and target element 27996.
Contact is detected due to initial settings.
Max. Geometric gap 6.054222446E-04 has been detected between contact
element 27959 and target element 27998.
*WARNING*: The geometric gap/penetration may be too large. Increase
pinball radius if it is a true geometric gap/penetration. Decrease
pinball if it is a false one.
****************************************
*** NOTE *** CP = 17.594 TIME= 09:30:02
Symmetric Deformable- deformable contact pair identified by real
constant set 118 and contact element type 117 has been set up. The
companion pair has real constant set ID 117. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.99659E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.19156E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.34841E-02
Average contact pair depth 0.19156E-02
Average target surface length 0.59569E-02
Default pinball region factor PINB 0.25000
*WARNING*: The default pinball radius may be too small to capture
contacting zone under small sliding assumption. Redefine the pinball
radius if necessary.
The resulting pinball region 0.47891E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.594 TIME= 09:30:02
No contact was detected for this contact pair.
****************************************
*** NOTE *** CP = 17.594 TIME= 09:30:02
Symmetric Deformable- deformable contact pair identified by real
constant set 119 and contact element type 119 has been set up. The
companion pair has real constant set ID 120. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.59135E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.67641E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.11896E-01
Average contact pair depth 0.67641E-02
Average target surface length 0.91805E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.16910E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.594 TIME= 09:30:02
Min. Initial gap 4.616567429E-04 was detected between contact element
28027 and target element 28062.
Contact is detected due to initial settings.
Max. Geometric gap 5.329327707E-04 has been detected between contact
element 28024 and target element 28058.
****************************************
*** NOTE *** CP = 17.594 TIME= 09:30:02
Symmetric Deformable- deformable contact pair identified by real
constant set 120 and contact element type 119 has been set up. The
companion pair has real constant set ID 119. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.59135E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.59744E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.94747E-02
Average contact pair depth 0.59744E-02
Average target surface length 0.11153E-01
Default pinball region factor PINB 0.25000
The resulting pinball region 0.14936E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.594 TIME= 09:30:02
Min. Initial gap 4.459523403E-04 was detected between contact element
28050 and target element 28007.
Contact is detected due to initial settings.
Max. Geometric gap 5.450774883E-04 has been detected between contact
element 28046 and target element 28004.
****************************************
*** NOTE *** CP = 17.594 TIME= 09:30:02
Symmetric Deformable- deformable contact pair identified by real
constant set 123 and contact element type 123 has been set up. The
companion pair has real constant set ID 124. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.52305E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.22691E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.36454E-02
Average contact pair depth 0.22691E-02
Average target surface length 0.12050E-01
Default pinball region factor PINB 0.25000
The resulting pinball region 0.56728E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.594 TIME= 09:30:02
Max. Initial penetration 6.938893904E-18 was detected between contact
element 28143 and target element 28170.
****************************************
*** NOTE *** CP = 17.594 TIME= 09:30:02
Symmetric Deformable- deformable contact pair identified by real
constant set 124 and contact element type 123 has been set up. The
companion pair has real constant set ID 123. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.52305E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.76475E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.12431E-01
Average contact pair depth 0.76475E-02
Average target surface length 0.37691E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.19119E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.594 TIME= 09:30:02
Max. Initial penetration 6.938893904E-18 was detected between contact
element 28159 and target element 28134.
****************************************
*** NOTE *** CP = 17.594 TIME= 09:30:02
Symmetric Deformable- deformable contact pair identified by real
constant set 125 and contact element type 125 has been set up. The
companion pair has real constant set ID 126. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.66769E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.24261E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.36454E-02
Average contact pair depth 0.24261E-02
Average target surface length 0.11869E-01
Default pinball region factor PINB 0.25000
The resulting pinball region 0.60652E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.594 TIME= 09:30:02
Max. Initial penetration 6.938893904E-18 was detected between contact
element 28199 and target element 28219.
****************************************
*** NOTE *** CP = 17.594 TIME= 09:30:02
Symmetric Deformable- deformable contact pair identified by real
constant set 126 and contact element type 125 has been set up. The
companion pair has real constant set ID 125. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.66769E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.59908E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.12246E-01
Average contact pair depth 0.59908E-02
Average target surface length 0.37691E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.14977E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.594 TIME= 09:30:02
Max. Initial penetration 6.938893904E-18 was detected between contact
element 28205 and target element 28186.
****************************************
*** NOTE *** CP = 17.594 TIME= 09:30:02
Symmetric Deformable- deformable contact pair identified by real
constant set 127 and contact element type 127 has been set up. The
companion pair has real constant set ID 128. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.48217E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.22750E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.36454E-02
Average contact pair depth 0.22750E-02
Average target surface length 0.10279E-01
Default pinball region factor PINB 0.25000
The resulting pinball region 0.56876E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.594 TIME= 09:30:02
Max. Initial penetration 1.736955052E-04 was detected between contact
element 28253 and target element 28272.
You may move entire target surface by : x= -8.197647376E-20, y=
-1.736955052E-04, z= -5.340808408E-20,to reduce initial penetration.
****************************************
*** NOTE *** CP = 17.594 TIME= 09:30:02
Symmetric Deformable- deformable contact pair identified by real
constant set 128 and contact element type 127 has been set up. The
companion pair has real constant set ID 127. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.48217E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.82959E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.10684E-01
Average contact pair depth 0.82959E-02
Average target surface length 0.37691E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.20740E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.594 TIME= 09:30:02
Max. Initial penetration 6.195620121E-05 was detected between contact
element 28255 and target element 28241.
You may move entire target surface by : x= 8.98413284E-06, y=
6.129957E-05, z= 4.679138581E-07,to reduce initial penetration.
****************************************
*** NOTE *** CP = 17.594 TIME= 09:30:02
Symmetric Deformable- deformable contact pair identified by real
constant set 129 and contact element type 129 has been set up. The
companion pair has real constant set ID 130. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.59273E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.24261E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.36454E-02
Average contact pair depth 0.24261E-02
Average target surface length 0.12056E-01
Default pinball region factor PINB 0.25000
The resulting pinball region 0.60652E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.594 TIME= 09:30:02
Max. Initial penetration 2.775557562E-17 was detected between contact
element 28305 and target element 28329.
****************************************
*** NOTE *** CP = 17.594 TIME= 09:30:02
Symmetric Deformable- deformable contact pair identified by real
constant set 130 and contact element type 129 has been set up. The
companion pair has real constant set ID 129. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.59273E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.67485E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.12438E-01
Average contact pair depth 0.67485E-02
Average target surface length 0.37691E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.16871E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.594 TIME= 09:30:02
Max. Initial penetration 5.551115123E-17 was detected between contact
element 28315 and target element 28293.
****************************************
*** NOTE *** CP = 17.594 TIME= 09:30:02
Symmetric Deformable- deformable contact pair identified by real
constant set 133 and contact element type 133 has been set up. The
companion pair has real constant set ID 134. Both pairs should have
the same behavior.
ANSYS will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.93276E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.42884E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.45003E-02
Average contact pair depth 0.42884E-02
Average target surface length 0.28005E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.10721E-02
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.594 TIME= 09:30:02
Min. Initial gap 4.890477239E-04 was detected between contact element
28820 and target element 28896.
Contact is detected due to initial settings.
Max. Geometric gap 5.056590656E-04 has been detected between contact
element 28812 and target element 28891.
****************************************
*** NOTE *** CP = 17.594 TIME= 09:30:02
Symmetric Deformable- deformable contact pair identified by real
constant set 134 and contact element type 133 has been set up. The
companion pair has real constant set ID 133. Both pairs should have
the same behavior.
ANSYS will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Linear contact is defined
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.93276E+16
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.21278E-03
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 0.28894E-02
Average contact pair depth 0.21278E-02
Average target surface length 0.43589E-02
Default pinball region factor PINB 0.25000
The resulting pinball region 0.53196E-03
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 17.594 TIME= 09:30:02
Min. Initial gap 4.900971609E-04 was detected between contact element
28862 and target element 28755.
Contact is detected due to initial settings.
Max. Geometric gap 5.07768796E-04 has been detected between contact
element 28856 and target element 28765.
*WARNING*: The geometric gap/penetration may be too large. Increase
pinball radius if it is a true geometric gap/penetration. Decrease
pinball if it is a false one.
****************************************
*WARNING*: The geometrical gap for certain contact pairs (e.g. ID 56)
may be too large. Increase pinball if it is a true geometrical gap.
Decrease pinball if it is a false one.
*************************************************
SUMMARY FOR CONTACT PAIR IDENTIFIED BY REAL CONSTANT SET 55
*** NOTE *** CP = 19.344 TIME= 09:30:03
Contact pair is inactive.
*************************************************
SUMMARY FOR CONTACT PAIR IDENTIFIED BY REAL CONSTANT SET 56
Max. Penetration of -1.683132053E-17 has been detected between contact
element 24675 and target element 24462.
Max. Geometrical gap of 5.103830541E-04 has been detected between
contact element 24711 and target element 24504.
*WARNING*: The geometrical gap may be too large. Increase pinball if
it is a true geometrical gap. Decrease pinball if it is a false one.
For total 94 contact elements, there are 44 elements are in contact.
There are 44 elements are in sticking.
Contacting area 1.020843316E-04.
Max. Pinball distance 5.538828809E-04.
One of the contact searching regions contains at least 22 target
elements.
Max. Pressure/force -3.46922569.
Max. Normal stiffness 1.759623794E+16.
Min. Normal stiffness 1.759623794E+16.
Max. Tangential stiffness 9.078842251E+15.
Min. Tangential stiffness 9.078842251E+15.
*************************************************
*************************************************
SUMMARY FOR CONTACT PAIR IDENTIFIED BY REAL CONSTANT SET 57
*** NOTE *** CP = 19.344 TIME= 09:30:03
Contact pair is inactive.
*************************************************
SUMMARY FOR CONTACT PAIR IDENTIFIED BY REAL CONSTANT SET 58
Max. Penetration of -1.536555953E-17 has been detected between contact
element 24904 and target element 24829.
Max. Geometrical gap of 5.061138968E-04 has been detected between
contact element 24909 and target element 24836.
*WARNING*: The geometrical gap may be too large. Increase pinball if
it is a true geometrical gap. Decrease pinball if it is a false one.
For total 33 contact elements, there are 33 elements are in contact.
There are 33 elements are in sticking.
Contacting area 1.020835468E-04.
Max. Pinball distance 6.333571329E-04.
One of the contact searching regions contains at least 11 target
elements.
Max. Pressure/force -7.49757121.
Max. Normal stiffness 1.020099076E+16.
Min. Normal stiffness 1.020099076E+16.
Max. Tangential stiffness 6.497823499E+15.
Min. Tangential stiffness 6.497823499E+15.
*************************************************
*************************************************
SUMMARY FOR CONTACT PAIR IDENTIFIED BY REAL CONSTANT SET 61
Max. Penetration of -1.682230688E-17 has been detected between contact
element 25260 and target element 25299.
Max. Geometrical gap of 6.188006116E-04 has been detected between
contact element 25275 and target element 25298.
*WARNING*: The geometrical gap may be too large. Increase pinball if
it is a true geometrical gap. Decrease pinball if it is a false one.
For total 84 contact elements, there are 43 elements are in contact.
There are 43 elements are in sticking.
Contacting area 6.459842046E-04.
Max. Pinball distance 8.307281584E-04.
One of the contact searching regions contains at least 6 target
elements.
Max. Pressure/force -3.60361718.
Max. Normal stiffness 5.115179205E+15.
Min. Normal stiffness 5.115179205E+15.
Max. Tangential stiffness 2.600463609E+15.
Min. Tangential stiffness 2.600463609E+15.
*************************************************
*************************************************
SUMMARY FOR CONTACT PAIR IDENTIFIED BY REAL CONSTANT SET 62
*** NOTE *** CP = 19.344 TIME= 09:30:03
Contact pair is inactive.
*************************************************
SUMMARY FOR CONTACT PAIR IDENTIFIED BY REAL CONSTANT SET 65
Max. Penetration of -3.469446952E-18 has been detected between contact
element 25360 and target element 25394.
Max. Geometrical penetration of -1.736955052E-04 has been detected
between contact element 25361 and target element 25388.
For total 12 contact elements, there are 12 elements are in contact.
There are 12 elements are in sticking.
Contacting area 1.598037028E-04.
Max. Pinball distance 5.687557171E-04.
One of the contact searching regions contains at least 10 target
elements.
Max. Pressure/force 1.659168649E-02.
Max. Normal stiffness 4.782228038E+15.
Min. Normal stiffness 4.782228038E+15.
Max. Tangential stiffness 2.238392511E+15.
Min. Tangential stiffness 2.238392511E+15.
*************************************************
*************************************************
SUMMARY FOR CONTACT PAIR IDENTIFIED BY REAL CONSTANT SET 66
*** NOTE *** CP = 19.344 TIME= 09:30:03
Contact pair is inactive.
*************************************************
SUMMARY FOR CONTACT PAIR IDENTIFIED BY REAL CONSTANT SET 69
Max. Penetration of -2.775557562E-17 has been detected between contact
element 25490 and target element 25531.
Max. Geometrical penetration of -1.021405789E-04 has been detected
between contact element 25492 and target element 25546.
For total 17 contact elements, there are 17 elements are in contact.
There are 17 elements are in sticking.
Contacting area 3.198795575E-04.
Max. Pinball distance 6.025809391E-04.
One of the contact searching regions contains at least 13 target
elements.
Max. Pressure/force 0.10475738.
Max. Normal stiffness 3.774282378E+15.
Min. Normal stiffness 3.774282378E+15.
Max. Tangential stiffness 1.00757777E+15.
Min. Tangential stiffness 1.00757777E+15.
*************************************************
*************************************************
SUMMARY FOR CONTACT PAIR IDENTIFIED BY REAL CONSTANT SET 70
*** NOTE *** CP = 19.344 TIME= 09:30:03
Contact pair is inactive.
*************************************************
SUMMARY FOR CONTACT PAIR IDENTIFIED BY REAL CONSTANT SET 73
Max. Penetration of -2.775557562E-17 has been detected between contact
element 25622 and target element 25651.
Max. Geometrical penetration of -1.736954878E-04 has been detected
between contact element 25627 and target element 25656.
For total 12 contact elements, there are 12 elements are in contact.
There are 12 elements are in sticking.
Contacting area 1.598037028E-04.
Max. Pinball distance 5.462957058E-04.
One of the contact searching regions contains at least 10 target
elements.
Max. Pressure/force -0.134715639.
Max. Normal stiffness 4.853642405E+15.
Min. Normal stiffness 4.853642405E+15.
Max. Tangential stiffness 2.182105532E+15.
Min. Tangential stiffness 2.182105532E+15.
*************************************************
*************************************************
SUMMARY FOR CONTACT PAIR IDENTIFIED BY REAL CONSTANT SET 74
*** NOTE *** CP = 19.344 TIME= 09:30:03
Contact pair is inactive.
*************************************************
SUMMARY FOR CONTACT PAIR IDENTIFIED BY REAL CONSTANT SET 75
*** NOTE *** CP = 19.344 TIME= 09:30:03
Contact pair is inactive.
*************************************************
SUMMARY FOR CONTACT PAIR IDENTIFIED BY REAL CONSTANT SET 76
*** NOTE *** CP = 19.344 TIME= 09:30:03
No contact was detected.
Max. Pinball distance 4.789121561E-04.
One of the contact searching regions contains at least 10 target
elements.
*************************************************
*************************************************
SUMMARY FOR CONTACT PAIR IDENTIFIED BY REAL CONSTANT SET 81
*** NOTE *** CP = 19.344 TIME= 09:30:03
Contact pair is inactive.
*************************************************
SUMMARY FOR CONTACT PAIR IDENTIFIED BY REAL CONSTANT SET 82
Max. Penetration of -1.388210579E-17 has been detected between contact
element 25925 and target element 25890.
Max. Geometrical gap of 6.261542594E-04 has been detected between
contact element 25925 and target element 25884.
For total 12 contact elements, there are 12 elements are in contact.
There are 12 elements are in sticking.
Contacting area 3.655751766E-04.
Max. Pinball distance 1.660523857E-03.
One of the contact searching regions contains at least 18 target
elements.
Max. Pressure/force -9.27171305.
Max. Normal stiffness 6.022195924E+15.
Min. Normal stiffness 6.022195924E+15.
Max. Tangential stiffness 3.371969748E+15.
Min. Tangential stiffness 3.371969748E+15.
*************************************************
*************************************************
SUMMARY FOR CONTACT PAIR IDENTIFIED BY REAL CONSTANT SET 83
Max. Penetration of -2.775557562E-17 has been detected between contact
element 25968 and target element 25993.
Max. Geometrical gap of 5.551115123E-17 has been detected between
contact element 25962 and target element 25989.
For total 12 contact elements, there are 12 elements are in contact.
There are 12 elements are in sticking.
Contacting area 1.598037028E-04.
Max. Pinball distance 5.672820508E-04.
One of the contact searching regions contains at least 7 target
elements.
Max. Pressure/force -0.16857417.
Max. Normal stiffness 6.073524563E+15.
Min. Normal stiffness 6.073524563E+15.
Max. Tangential stiffness 2.835437129E+15.
Min. Tangential stiffness 2.835437129E+15.
*************************************************
*************************************************
SUMMARY FOR CONTACT PAIR IDENTIFIED BY REAL CONSTANT SET 84
*** NOTE *** CP = 19.344 TIME= 09:30:03
Contact pair is inactive.
*************************************************
SUMMARY FOR CONTACT PAIR IDENTIFIED BY REAL CONSTANT SET 85
Max. Penetration of -2.775557562E-17 has been detected between contact
element 26012 and target element 26037.
Max. Geometrical gap of 5.551115123E-17 has been detected between
contact element 26009 and target element 26050.
Max. Geometrical penetration of -1.736954878E-04 has been detected
between contact element 26009 and target element 26050.
For total 12 contact elements, there are 12 elements are in contact.
There are 12 elements are in sticking.
Contacting area 1.598037028E-04.
Max. Pinball distance 5.687557171E-04.
One of the contact searching regions contains at least 10 target
elements.
Max. Pressure/force -0.148170475.
Max. Normal stiffness 5.338403957E+15.
Min. Normal stiffness 5.338403957E+15.
Max. Tangential stiffness 2.498718869E+15.
Min. Tangential stiffness 2.498718869E+15.
*************************************************
*************************************************
SUMMARY FOR CONTACT PAIR IDENTIFIED BY REAL CONSTANT SET 86
*** NOTE *** CP = 19.344 TIME= 09:30:03
Contact pair is inactive.
*************************************************
SUMMARY FOR CONTACT PAIR IDENTIFIED BY REAL CONSTANT SET 87
*** NOTE *** CP = 19.344 TIME= 09:30:03
Contact pair is inactive.
*************************************************
SUMMARY FOR CONTACT PAIR IDENTIFIED BY REAL CONSTANT SET 88
Max. Penetration of -8.696400537E-19 has been detected between contact
element 26127 and target element 26073.
Max. Geometrical gap of 6.001E-04 has been detected between contact
element 26117 and target element 26061.
*WARNING*: The geometrical gap may be too large. Increase pinball if
it is a true geometrical gap. Decrease pinball if it is a false one.
For total 12 contact elements, there are 12 elements are in contact.
There are 12 elements are in sticking.
Contacting area 6.88562182E-05.
Max. Pinball distance 6.04807593E-04.
One of the contact searching regions contains at least 14 target
elements.
Max. Pressure/force -4.59686777.
Max. Normal stiffness 9.459454222E+15.
Min. Normal stiffness 9.459454222E+15.
Max. Tangential stiffness 4.770937995E+15.
Min. Tangential stiffness 4.770937995E+15.
*************************************************
*************************************************
SUMMARY FOR CONTACT PAIR IDENTIFIED BY REAL CONSTANT SET 93
*** NOTE *** CP = 19.344 TIME= 09:30:03
Contact pair is inactive.
*************************************************
SUMMARY FOR CONTACT PAIR IDENTIFIED BY REAL CONSTANT SET 94
Max. Penetration of -5.225028234E-17 has been detected between contact
element 26545 and target element 26332.
Max. Geometrical gap of 5.057185782E-04 has been detected between
contact element 26554 and target element 26357.
*WARNING*: The geometrical gap may be too large. Increase pinball if
it is a true geometrical gap. Decrease pinball if it is a false one.
For total 95 contact elements, there are 44 elements are in contact.
There are 44 elements are in sticking.
Contacting area 1.020843317E-04.
Max. Pinball distance 5.542362277E-04.
One of the contact searching regions contains at least 18 target
elements.
Max. Pressure/force -5.06575065.
Max. Normal stiffness 1.742895099E+16.
Min. Normal stiffness 1.742895099E+16.
Max. Tangential stiffness 9.031742714E+15.
Min. Tangential stiffness 9.031742714E+15.
*************************************************
*************************************************
SUMMARY FOR CONTACT PAIR IDENTIFIED BY REAL CONSTANT SET 97
Max. Penetration of -5.551115123E-17 has been detected between contact
element 26852 and target element 26886.
For total 17 contact elements, there are 17 elements are in contact.
There are 17 elements are in sticking.
Contacting area 1.882075331E-04.
Max. Pinball distance 6.107597346E-04.
One of the contact searching regions contains at least 7 target
elements.
Max. Pressure/force 0.172825094.
Max. Normal stiffness 3.113340108E+15.
Min. Normal stiffness 3.113340108E+15.
Max. Tangential stiffness 8.424143619E+14.
Min. Tangential stiffness 8.424143619E+14.
*************************************************
*************************************************
SUMMARY FOR CONTACT PAIR IDENTIFIED BY REAL CONSTANT SET 98
*** NOTE *** CP = 19.344 TIME= 09:30:03
Contact pair is inactive.
*************************************************
SUMMARY FOR CONTACT PAIR IDENTIFIED BY REAL CONSTANT SET 105
Max. Penetration of -5.551115123E-17 has been detected between contact
element 27517 and target element 27550.
For total 17 contact elements, there are 17 elements are in contact.
There are 17 elements are in sticking.
Contacting area 1.882075321E-04.
Max. Pinball distance 5.995155631E-04.
One of the contact searching regions contains at least 7 target
elements.
Max. Pressure/force 0.186985235.
Max. Normal stiffness 3.368426542E+15.
Min. Normal stiffness 3.368426542E+15.
Max. Tangential stiffness 8.94656539E+14.
Min. Tangential stiffness 8.94656539E+14.
*************************************************
*************************************************
SUMMARY FOR CONTACT PAIR IDENTIFIED BY REAL CONSTANT SET 106
*** NOTE *** CP = 19.344 TIME= 09:30:03
Contact pair is inactive.
*************************************************
SUMMARY FOR CONTACT PAIR IDENTIFIED BY REAL CONSTANT SET 113
*** NOTE *** CP = 19.344 TIME= 09:30:03
Contact pair is inactive.
*************************************************
SUMMARY FOR CONTACT PAIR IDENTIFIED BY REAL CONSTANT SET 114
Max. Penetration of -1.107908108E-17 has been detected between contact
element 27816 and target element 27781.
Max. Geometrical gap of 1.388433726E-03 has been detected between
contact element 27816 and target element 27781.
*WARNING*: The geometrical gap may be too large. Increase pinball if
it is a true geometrical gap. Decrease pinball if it is a false one.
For total 12 contact elements, there are 12 elements are in contact.
There are 12 elements are in sticking.
Contacting area 3.441695228E-04.
Max. Pinball distance 1.502046115E-03.
One of the contact searching regions contains at least 17 target
elements.
Max. Pressure/force -5.34206213.
Max. Normal stiffness 6.148976656E+15.
Min. Normal stiffness 6.148976656E+15.
Max. Tangential stiffness 2.922845955E+15.
Min. Tangential stiffness 2.922845955E+15.
*************************************************
*************************************************
SUMMARY FOR CONTACT PAIR IDENTIFIED BY REAL CONSTANT SET 115
*** NOTE *** CP = 19.344 TIME= 09:30:03
Contact pair is inactive.
*************************************************
SUMMARY FOR CONTACT PAIR IDENTIFIED BY REAL CONSTANT SET 116
Max. Penetration of -5.43189669E-18 has been detected between contact
element 27891 and target element 27855.
Max. Geometrical gap of 5.876189201E-04 has been detected between
contact element 27883 and target element 27844.
For total 13 contact elements, there are 13 elements are in contact.
There are 13 elements are in sticking.
Contacting area 3.673304724E-04.
Max. Pinball distance 1.506707746E-03.
One of the contact searching regions contains at least 16 target
elements.
Max. Pressure/force -8.18224407.
Max. Normal stiffness 4.782482946E+15.
Min. Normal stiffness 4.782482946E+15.
Max. Tangential stiffness 2.373758172E+15.
Min. Tangential stiffness 2.373758172E+15.
*************************************************
*************************************************
SUMMARY FOR CONTACT PAIR IDENTIFIED BY REAL CONSTANT SET 117
*** NOTE *** CP = 19.344 TIME= 09:30:03
Contact pair is inactive.
*************************************************
SUMMARY FOR CONTACT PAIR IDENTIFIED BY REAL CONSTANT SET 118
*** NOTE *** CP = 19.344 TIME= 09:30:03
No contact was detected.
Max. Pinball distance 4.789121562E-04.
One of the contact searching regions contains at least 11 target
elements.
*************************************************
*************************************************
SUMMARY FOR CONTACT PAIR IDENTIFIED BY REAL CONSTANT SET 119
*** NOTE *** CP = 19.344 TIME= 09:30:03
Contact pair is inactive.
*************************************************
SUMMARY FOR CONTACT PAIR IDENTIFIED BY REAL CONSTANT SET 120
Max. Penetration of -2.45709621E-17 has been detected between contact
element 28040 and target element 28009.
Max. Geometrical gap of 5.450774883E-04 has been detected between
contact element 28046 and target element 28004.
For total 12 contact elements, there are 12 elements are in contact.
There are 12 elements are in sticking.
Contacting area 3.664891599E-04.
Max. Pinball distance 1.493604424E-03.
One of the contact searching regions contains at least 16 target
elements.
Max. Pressure/force -4.48446301.
Max. Normal stiffness 5.913531869E+15.
Min. Normal stiffness 5.913531869E+15.
Max. Tangential stiffness 2.796661541E+15.
Min. Tangential stiffness 2.796661541E+15.
*************************************************
*************************************************
SUMMARY FOR CONTACT PAIR IDENTIFIED BY REAL CONSTANT SET 123
Max. Penetration of -3.469446952E-18 has been detected between contact
element 28145 and target element 28176.
Max. Geometrical penetration of -6.938893904E-18 has been detected
between contact element 28143 and target element 28170.
For total 12 contact elements, there are 12 elements are in contact.
There are 12 elements are in sticking.
Contacting area 1.598037028E-04.
Max. Pinball distance 5.672820508E-04.
One of the contact searching regions contains at least 7 target
elements.
Max. Pressure/force -1.814687823E-02.
Max. Normal stiffness 5.230481538E+15.
Min. Normal stiffness 5.230481538E+15.
Max. Tangential stiffness 2.441860802E+15.
Min. Tangential stiffness 2.441860802E+15.
*************************************************
*************************************************
SUMMARY FOR CONTACT PAIR IDENTIFIED BY REAL CONSTANT SET 124
*** NOTE *** CP = 19.344 TIME= 09:30:03
Contact pair is inactive.
*************************************************
SUMMARY FOR CONTACT PAIR IDENTIFIED BY REAL CONSTANT SET 125
Max. Penetration of -1.34916888E-32 has been detected between contact
element 28202 and target element 28227.
Max. Geometrical gap of 3.469446952E-18 has been detected between
contact element 28199 and target element 28219.
Max. Geometrical penetration of -6.938893904E-18 has been detected
between contact element 28199 and target element 28219.
For total 12 contact elements, there are 12 elements are in contact.
There are 12 elements are in sticking.
Contacting area 1.598037028E-04.
Max. Pinball distance 6.065243642E-04.
One of the contact searching regions contains at least 9 target
elements.
Max. Pressure/force -2.316525445E-02.
Max. Normal stiffness 6.676930005E+15.
Min. Normal stiffness 6.676930005E+15.
Max. Tangential stiffness 3.332769377E+15.
Min. Tangential stiffness 3.332769377E+15.
*************************************************
*************************************************
SUMMARY FOR CONTACT PAIR IDENTIFIED BY REAL CONSTANT SET 126
*** NOTE *** CP = 19.344 TIME= 09:30:03
Contact pair is inactive.
*************************************************
SUMMARY FOR CONTACT PAIR IDENTIFIED BY REAL CONSTANT SET 127
Max. Penetration of -3.469446952E-18 has been detected between contact
element 28244 and target element 28285.
Max. Geometrical penetration of -1.736955052E-04 has been detected
between contact element 28253 and target element 28272.
For total 12 contact elements, there are 12 elements are in contact.
There are 12 elements are in sticking.
Contacting area 1.598037028E-04.
Max. Pinball distance 5.687557171E-04.
One of the contact searching regions contains at least 8 target
elements.
Max. Pressure/force 1.672851227E-02.
Max. Normal stiffness 4.821665384E+15.
Min. Normal stiffness 4.821665384E+15.
Max. Tangential stiffness 2.256851743E+15.
Min. Tangential stiffness 2.256851743E+15.
*************************************************
*************************************************
SUMMARY FOR CONTACT PAIR IDENTIFIED BY REAL CONSTANT SET 128
*** NOTE *** CP = 19.344 TIME= 09:30:03
Contact pair is inactive.
*************************************************
SUMMARY FOR CONTACT PAIR IDENTIFIED BY REAL CONSTANT SET 129
Max. Penetration of -2.775557562E-17 has been detected between contact
element 28304 and target element 28328.
Max. Geometrical gap of 5.551115123E-17 has been detected between
contact element 28310 and target element 28334.
For total 12 contact elements, there are 12 elements are in contact.
There are 12 elements are in sticking.
Contacting area 1.598037028E-04.
Max. Pinball distance 6.065243642E-04.
One of the contact searching regions contains at least 9 target
elements.
Max. Pressure/force -0.164514472.
Max. Normal stiffness 5.927258501E+15.
Min. Normal stiffness 5.927258501E+15.
Max. Tangential stiffness 2.958573118E+15.
Min. Tangential stiffness 2.958573118E+15.
*************************************************
*************************************************
SUMMARY FOR CONTACT PAIR IDENTIFIED BY REAL CONSTANT SET 130
*** NOTE *** CP = 19.344 TIME= 09:30:03
Contact pair is inactive.
*************************************************
SUMMARY FOR CONTACT PAIR IDENTIFIED BY REAL CONSTANT SET 133
*** NOTE *** CP = 19.344 TIME= 09:30:03
Contact pair is inactive.
*************************************************
SUMMARY FOR CONTACT PAIR IDENTIFIED BY REAL CONSTANT SET 134
Max. Penetration of -2.30930359E-17 has been detected between contact
element 28851 and target element 28773.
Max. Geometrical gap of 5.07768796E-04 has been detected between
contact element 28856 and target element 28765.
*WARNING*: The geometrical gap may be too large. Increase pinball if
it is a true geometrical gap. Decrease pinball if it is a false one.
For total 35 contact elements, there are 35 elements are in contact.
There are 35 elements are in sticking.
Contacting area 1.02084498E-04.
Max. Pinball distance 5.319597865E-04.
One of the contact searching regions contains at least 10 target
elements.
Max. Pressure/force -0.401954121.
Max. Normal stiffness 9.327583583E+15.
Min. Normal stiffness 9.327583583E+15.
Max. Tangential stiffness 5.151796179E+15.
Min. Tangential stiffness 5.151796179E+15.
*************************************************
*********** PRECISE MASS SUMMARY ***********
TOTAL RIGID BODY MASS MATRIX ABOUT ORIGIN
Translational mass | Coupled translational/rotational mass
11.812 0.0000 0.0000 | 0.0000 0.41770 -1.1361
0.0000 11.812 0.0000 | -0.41770 0.0000 0.51568
0.0000 0.0000 11.812 | 1.1361 -0.51568 0.0000
------------------------------------------ | ------------------------------------------
| Rotational mass (inertia)
| 0.23035 -0.46411E-01 -0.17530E-01
| -0.46411E-01 0.11326 -0.40289E-01
| -0.17530E-01 -0.40289E-01 0.30347
TOTAL MASS = 11.812
The mass principal axes coincide with the global Cartesian axes
CENTER OF MASS (X,Y,Z)= 0.43658E-01 0.96179E-01 0.35362E-01
TOTAL INERTIA ABOUT CENTER OF MASS
0.10631 0.31866E-02 0.70622E-03
0.31866E-02 0.75980E-01 -0.11569E-03
0.70622E-03 -0.11569E-03 0.17169
PRINCIPAL INERTIAS = 0.10664 0.75648E-01 0.17170
ORIENTATION VECTORS OF THE INERTIA PRINCIPAL AXES IN GLOBAL CARTESIAN
( 0.995, 0.103,-0.011) (-0.103, 0.995, 0.002) ( 0.011,-0.001, 1.000)
*** MASS SUMMARY BY ELEMENT TYPE ***
TYPE MASS
1 0.151602E-01
2 0.641873E-01
3 0.109171E-03
4 0.302682E-02
5 0.627379E-02
6 0.627413E-02
7 0.151488E-01
8 0.163757E-03
9 0.297215E-02
10 0.759495E-02
11 0.214954E-03
12 0.319532E-01
13 0.463410E-04
14 0.984632E-04
15 0.303752E-02
16 0.109171E-03
17 0.302682E-02
18 0.767462E-02
19 0.133227E-03
20 0.315946E-01
21 0.692437E-04
22 0.734478E-02
23 0.343346E-03
24 0.646326E-01
25 0.627391E-02
26 0.627416E-02
27 0.151482E-01
28 0.646270E-01
29 0.627391E-02
30 0.627416E-02
31 0.151527E-01
32 0.316388E-01
33 0.625935E-04
34 0.321521E-01
35 0.463410E-04
36 0.984632E-04
37 0.303752E-02
38 0.313615E-02
39 0.109171E-03
40 0.302682E-02
41 0.756194E-02
42 0.154716E-03
43 0.313615E-02
44 0.645310E-01
45 0.627379E-02
46 0.627405E-02
47 1.63179
48 5.42786
49 0.957772
50 0.526529E-01
51 0.964794
52 0.459709E-01
53 1.08935
54 1.08935
Range of element maximum matrix coefficients in global coordinates
Maximum = 8.366889911E+10 at element 26256.
Minimum = 106409364 at element 8907.
*** ELEMENT MATRIX FORMULATION TIMES
TYPE NUMBER ENAME TOTAL CP AVE CP
1 300 SOLID187 0.078 0.000260
2 1276 SOLID187 0.188 0.000147
3 12 SOLID187 0.000 0.000000
4 18 SOLID186 0.000 0.000000
5 190 SOLID187 0.016 0.000082
6 120 SOLID187 0.016 0.000130
7 293 SOLID187 0.062 0.000213
8 18 SOLID187 0.000 0.000000
9 21 SOLID186 0.000 0.000000
10 119 SOLID187 0.016 0.000131
11 3 SOLID186 0.000 0.000000
12 256 SOLID187 0.031 0.000122
13 4 SOLID186 0.000 0.000000
14 12 SOLID187 0.000 0.000000
15 18 SOLID186 0.000 0.000000
16 12 SOLID187 0.000 0.000000
17 18 SOLID186 0.016 0.000868
18 153 SOLID187 0.031 0.000204
19 4 SOLID186 0.000 0.000000
20 233 SOLID187 0.031 0.000134
21 6 SOLID186 0.000 0.000000
22 124 SOLID187 0.031 0.000252
23 4 SOLID186 0.000 0.000000
24 1219 SOLID187 0.062 0.000051
25 192 SOLID187 0.031 0.000163
26 122 SOLID187 0.016 0.000128
27 279 SOLID187 0.000 0.000000
28 1236 SOLID187 0.266 0.000215
29 193 SOLID187 0.031 0.000162
30 122 SOLID187 0.016 0.000128
31 278 SOLID187 0.078 0.000281
32 214 SOLID187 0.031 0.000146
33 6 SOLID186 0.000 0.000000
34 265 SOLID187 0.062 0.000236
35 4 SOLID186 0.000 0.000000
36 12 SOLID187 0.000 0.000000
37 18 SOLID186 0.016 0.000868
38 12 SOLID186 0.000 0.000000
39 12 SOLID187 0.016 0.001302
40 18 SOLID186 0.000 0.000000
41 151 SOLID187 0.016 0.000103
42 4 SOLID186 0.000 0.000000
43 12 SOLID186 0.000 0.000000
44 1281 SOLID187 0.188 0.000146
45 190 SOLID187 0.031 0.000164
46 127 SOLID187 0.000 0.000000
47 562 SOLID187 0.094 0.000167
48 2815 SOLID187 0.359 0.000128
49 464 SOLID187 0.094 0.000202
50 20 SOLID186 0.000 0.000000
51 512 SOLID187 0.094 0.000183
52 20 SOLID186 0.000 0.000000
53 490 SOLID186 0.078 0.000159
54 390 SOLID186 0.094 0.000240
55 198 CONTA174 0.000 0.000000
56 198 TARGE170 0.000 0.000000
57 76 CONTA174 0.016 0.000206
58 76 TARGE170 0.000 0.000000
59 77 CONTA174 0.047 0.000609
60 77 TARGE170 0.016 0.000203
61 96 CONTA174 0.031 0.000326
62 96 TARGE170 0.000 0.000000
63 21 CONTA174 0.000 0.000000
64 21 TARGE170 0.000 0.000000
65 28 CONTA174 0.000 0.000000
66 28 TARGE170 0.000 0.000000
67 33 CONTA174 0.000 0.000000
68 33 TARGE170 0.000 0.000000
69 43 CONTA174 0.000 0.000000
70 43 TARGE170 0.000 0.000000
71 24 CONTA174 0.000 0.000000
72 24 TARGE170 0.000 0.000000
73 29 CONTA174 0.000 0.000000
74 29 TARGE170 0.000 0.000000
75 48 CONTA174 0.016 0.000326
76 48 TARGE170 0.000 0.000000
77 27 CONTA174 0.000 0.000000
78 27 TARGE170 0.000 0.000000
79 33 CONTA174 0.000 0.000000
80 33 TARGE170 0.000 0.000000
81 34 CONTA174 0.000 0.000000
82 34 TARGE170 0.000 0.000000
83 25 CONTA174 0.016 0.000625
84 25 TARGE170 0.000 0.000000
85 29 CONTA174 0.000 0.000000
86 29 TARGE170 0.000 0.000000
87 43 CONTA174 0.000 0.000000
88 43 TARGE170 0.000 0.000000
89 33 CONTA174 0.000 0.000000
90 33 TARGE170 0.000 0.000000
91 36 CONTA174 0.000 0.000000
92 36 TARGE170 0.000 0.000000
93 198 CONTA174 0.016 0.000079
94 198 TARGE170 0.000 0.000000
95 75 CONTA174 0.016 0.000208
96 75 TARGE170 0.000 0.000000
97 32 CONTA174 0.000 0.000000
98 32 TARGE170 0.000 0.000000
99 33 CONTA174 0.000 0.000000
100 33 TARGE170 0.000 0.000000
101 197 CONTA174 0.062 0.000317
102 197 TARGE170 0.000 0.000000
103 75 CONTA174 0.000 0.000000
104 75 TARGE170 0.000 0.000000
105 32 CONTA174 0.016 0.000488
106 32 TARGE170 0.000 0.000000
107 33 CONTA174 0.000 0.000000
108 33 TARGE170 0.000 0.000000
109 40 CONTA174 0.000 0.000000
110 40 TARGE170 0.000 0.000000
111 27 CONTA174 0.000 0.000000
112 27 TARGE170 0.000 0.000000
113 38 CONTA174 0.016 0.000411
114 38 TARGE170 0.000 0.000000
115 34 CONTA174 0.000 0.000000
116 34 TARGE170 0.000 0.000000
117 47 CONTA174 0.000 0.000000
118 47 TARGE170 0.000 0.000000
119 32 CONTA174 0.000 0.000000
120 32 TARGE170 0.000 0.000000
121 33 CONTA174 0.016 0.000473
122 33 TARGE170 0.000 0.000000
123 25 CONTA174 0.000 0.000000
124 25 TARGE170 0.000 0.000000
125 25 CONTA174 0.016 0.000625
126 25 TARGE170 0.000 0.000000
127 29 CONTA174 0.000 0.000000
128 29 TARGE170 0.000 0.000000
129 25 CONTA174 0.000 0.000000
130 25 TARGE170 0.000 0.000000
131 204 CONTA174 0.016 0.000077
132 204 TARGE170 0.000 0.000000
133 82 CONTA174 0.016 0.000191
134 82 TARGE170 0.000 0.000000
135 84 CONTA174 0.000 0.000000
136 84 TARGE170 0.000 0.000000
137 108 CONTA174 0.016 0.000145
138 108 TARGE170 0.000 0.000000
139 33 CONTA174 0.000 0.000000
140 33 TARGE170 0.000 0.000000
141 42 CONTA174 0.016 0.000372
142 42 TARGE170 0.000 0.000000
143 770 SURF154 0.078 0.000101
Time at end of element matrix formulation CP = 19.34375.
DISTRIBUTED PCG SOLVER SOLUTION CONVERGED
DISTRIBUTED PCG SOLVER SOLUTION STATISTICS
NUMBER OF ITERATIONS= 417
NUMBER OF EQUATIONS = 92055
LEVEL OF CONVERGENCE= 1
CALCULATED NORM = 0.92531E-08
SPECIFIED TOLERANCE = 0.10000E-07
TOTAL CPU TIME (sec)= 4.55
TOTAL WALL TIME(sec)= 4.54
TOTAL MEMORY (GB) = 0.05
*** ELEMENT RESULT CALCULATION TIMES
TYPE NUMBER ENAME TOTAL CP AVE CP
1 300 SOLID187 0.031 0.000104
2 1276 SOLID187 0.109 0.000086
3 12 SOLID187 0.000 0.000000
4 18 SOLID186 0.000 0.000000
5 190 SOLID187 0.062 0.000329
6 120 SOLID187 0.031 0.000260
7 293 SOLID187 0.000 0.000000
8 18 SOLID187 0.000 0.000000
9 21 SOLID186 0.016 0.000744
10 119 SOLID187 0.031 0.000263
11 3 SOLID186 0.000 0.000000
12 256 SOLID187 0.016 0.000061
13 4 SOLID186 0.000 0.000000
14 12 SOLID187 0.016 0.001302
15 18 SOLID186 0.000 0.000000
16 12 SOLID187 0.016 0.001302
17 18 SOLID186 0.000 0.000000
18 153 SOLID187 0.016 0.000102
19 4 SOLID186 0.000 0.000000
20 233 SOLID187 0.062 0.000268
21 6 SOLID186 0.000 0.000000
22 124 SOLID187 0.016 0.000126
23 4 SOLID186 0.000 0.000000
24 1219 SOLID187 0.109 0.000090
25 192 SOLID187 0.016 0.000081
26 122 SOLID187 0.016 0.000128
27 279 SOLID187 0.016 0.000056
28 1236 SOLID187 0.156 0.000126
29 193 SOLID187 0.031 0.000162
30 122 SOLID187 0.031 0.000256
31 278 SOLID187 0.016 0.000056
32 214 SOLID187 0.000 0.000000
33 6 SOLID186 0.000 0.000000
34 265 SOLID187 0.047 0.000177
35 4 SOLID186 0.000 0.000000
36 12 SOLID187 0.000 0.000000
37 18 SOLID186 0.000 0.000000
38 12 SOLID186 0.000 0.000000
39 12 SOLID187 0.000 0.000000
40 18 SOLID186 0.000 0.000000
41 151 SOLID187 0.047 0.000310
42 4 SOLID186 0.000 0.000000
43 12 SOLID186 0.000 0.000000
44 1281 SOLID187 0.172 0.000134
45 190 SOLID187 0.062 0.000329
46 127 SOLID187 0.000 0.000000
47 562 SOLID187 0.062 0.000111
48 2815 SOLID187 0.281 0.000100
49 464 SOLID187 0.047 0.000101
50 20 SOLID186 0.000 0.000000
51 512 SOLID187 0.047 0.000092
52 20 SOLID186 0.016 0.000781
53 490 SOLID186 0.078 0.000159
54 390 SOLID186 0.047 0.000120
55 198 CONTA174 0.000 0.000000
57 76 CONTA174 0.000 0.000000
59 77 CONTA174 0.000 0.000000
61 96 CONTA174 0.000 0.000000
63 21 CONTA174 0.000 0.000000
65 28 CONTA174 0.000 0.000000
67 33 CONTA174 0.000 0.000000
69 43 CONTA174 0.000 0.000000
71 24 CONTA174 0.000 0.000000
73 29 CONTA174 0.000 0.000000
75 48 CONTA174 0.000 0.000000
77 27 CONTA174 0.000 0.000000
79 33 CONTA174 0.000 0.000000
81 34 CONTA174 0.000 0.000000
83 25 CONTA174 0.000 0.000000
85 29 CONTA174 0.000 0.000000
87 43 CONTA174 0.000 0.000000
89 33 CONTA174 0.000 0.000000
91 36 CONTA174 0.000 0.000000
93 198 CONTA174 0.016 0.000079
95 75 CONTA174 0.000 0.000000
97 32 CONTA174 0.000 0.000000
99 33 CONTA174 0.000 0.000000
101 197 CONTA174 0.016 0.000079
103 75 CONTA174 0.000 0.000000
105 32 CONTA174 0.016 0.000488
107 33 CONTA174 0.000 0.000000
109 40 CONTA174 0.016 0.000391
111 27 CONTA174 0.000 0.000000
113 38 CONTA174 0.000 0.000000
115 34 CONTA174 0.000 0.000000
117 47 CONTA174 0.000 0.000000
119 32 CONTA174 0.000 0.000000
121 33 CONTA174 0.000 0.000000
123 25 CONTA174 0.000 0.000000
125 25 CONTA174 0.000 0.000000
127 29 CONTA174 0.000 0.000000
129 25 CONTA174 0.000 0.000000
131 204 CONTA174 0.000 0.000000
133 82 CONTA174 0.000 0.000000
135 84 CONTA174 0.016 0.000186
137 108 CONTA174 0.000 0.000000
139 33 CONTA174 0.000 0.000000
141 42 CONTA174 0.000 0.000000
143 770 SURF154 0.031 0.000041
*** NODAL LOAD CALCULATION TIMES
TYPE NUMBER ENAME TOTAL CP AVE CP
1 300 SOLID187 0.000 0.000000
2 1276 SOLID187 0.047 0.000037
3 12 SOLID187 0.000 0.000000
4 18 SOLID186 0.000 0.000000
5 190 SOLID187 0.000 0.000000
6 120 SOLID187 0.000 0.000000
7 293 SOLID187 0.000 0.000000
8 18 SOLID187 0.000 0.000000
9 21 SOLID186 0.000 0.000000
10 119 SOLID187 0.000 0.000000
11 3 SOLID186 0.000 0.000000
12 256 SOLID187 0.000 0.000000
13 4 SOLID186 0.000 0.000000
14 12 SOLID187 0.000 0.000000
15 18 SOLID186 0.000 0.000000
16 12 SOLID187 0.000 0.000000
17 18 SOLID186 0.000 0.000000
18 153 SOLID187 0.000 0.000000
19 4 SOLID186 0.000 0.000000
20 233 SOLID187 0.000 0.000000
21 6 SOLID186 0.000 0.000000
22 124 SOLID187 0.000 0.000000
23 4 SOLID186 0.000 0.000000
24 1219 SOLID187 0.031 0.000026
25 192 SOLID187 0.016 0.000081
26 122 SOLID187 0.000 0.000000
27 279 SOLID187 0.016 0.000056
28 1236 SOLID187 0.047 0.000038
29 193 SOLID187 0.000 0.000000
30 122 SOLID187 0.000 0.000000
31 278 SOLID187 0.000 0.000000
32 214 SOLID187 0.016 0.000073
33 6 SOLID186 0.000 0.000000
34 265 SOLID187 0.000 0.000000
35 4 SOLID186 0.000 0.000000
36 12 SOLID187 0.000 0.000000
37 18 SOLID186 0.016 0.000868
38 12 SOLID186 0.000 0.000000
39 12 SOLID187 0.000 0.000000
40 18 SOLID186 0.000 0.000000
41 151 SOLID187 0.000 0.000000
42 4 SOLID186 0.000 0.000000
43 12 SOLID186 0.000 0.000000
44 1281 SOLID187 0.031 0.000024
45 190 SOLID187 0.016 0.000082
46 127 SOLID187 0.000 0.000000
47 562 SOLID187 0.031 0.000056
48 2815 SOLID187 0.062 0.000022
49 464 SOLID187 0.031 0.000067
50 20 SOLID186 0.000 0.000000
51 512 SOLID187 0.047 0.000092
52 20 SOLID186 0.000 0.000000
53 490 SOLID186 0.000 0.000000
54 390 SOLID186 0.016 0.000040
55 198 CONTA174 0.000 0.000000
57 76 CONTA174 0.000 0.000000
59 77 CONTA174 0.000 0.000000
61 96 CONTA174 0.000 0.000000
63 21 CONTA174 0.000 0.000000
65 28 CONTA174 0.000 0.000000
67 33 CONTA174 0.000 0.000000
69 43 CONTA174 0.000 0.000000
71 24 CONTA174 0.000 0.000000
73 29 CONTA174 0.000 0.000000
75 48 CONTA174 0.000 0.000000
77 27 CONTA174 0.000 0.000000
79 33 CONTA174 0.000 0.000000
81 34 CONTA174 0.000 0.000000
83 25 CONTA174 0.000 0.000000
85 29 CONTA174 0.000 0.000000
87 43 CONTA174 0.000 0.000000
89 33 CONTA174 0.000 0.000000
91 36 CONTA174 0.000 0.000000
93 198 CONTA174 0.000 0.000000
95 75 CONTA174 0.000 0.000000
97 32 CONTA174 0.000 0.000000
99 33 CONTA174 0.000 0.000000
101 197 CONTA174 0.000 0.000000
103 75 CONTA174 0.000 0.000000
105 32 CONTA174 0.000 0.000000
107 33 CONTA174 0.000 0.000000
109 40 CONTA174 0.000 0.000000
111 27 CONTA174 0.000 0.000000
113 38 CONTA174 0.000 0.000000
115 34 CONTA174 0.000 0.000000
117 47 CONTA174 0.000 0.000000
119 32 CONTA174 0.000 0.000000
121 33 CONTA174 0.000 0.000000
123 25 CONTA174 0.000 0.000000
125 25 CONTA174 0.000 0.000000
127 29 CONTA174 0.000 0.000000
129 25 CONTA174 0.000 0.000000
131 204 CONTA174 0.000 0.000000
133 82 CONTA174 0.016 0.000191
135 84 CONTA174 0.000 0.000000
137 108 CONTA174 0.000 0.000000
139 33 CONTA174 0.000 0.000000
141 42 CONTA174 0.000 0.000000
143 770 SURF154 0.016 0.000020
*** LOAD STEP 1 SUBSTEP 1 COMPLETED. CUM ITER = 1
*** TIME = 1.00000 TIME INC = 1.00000 NEW TRIANG MATRIX
*** MAPDL BINARY FILE STATISTICS
BUFFER SIZE USED= 16384
12.625 MB WRITTEN ON ELEMENT SAVED DATA FILE: file0.esav
7.375 MB WRITTEN ON RESULTS FILE: file0.rst
Plot equivalent stress#
Plot equivalent stress.
mapdl.post1() # Enter postprocessor
mapdl.set(1, 1) # Select first load step
mapdl.post_processing.plot_nodal_eqv_stress() # Plot equivalent stress
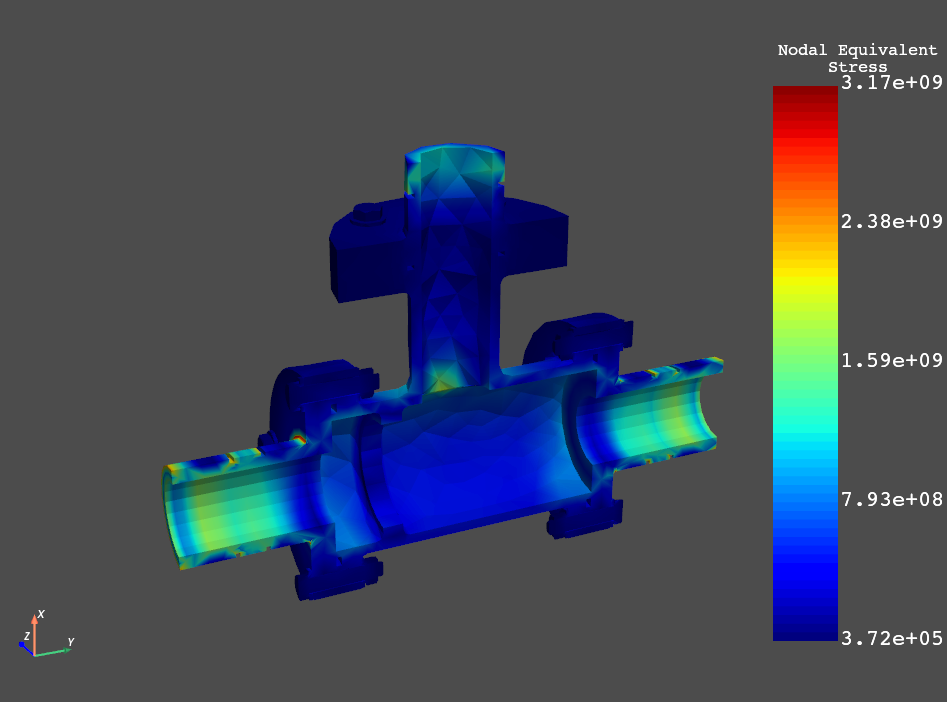
Exit MAPDL instance#
Exit the MAPDL instance.
mapdl.exit()
Total running time of the script: ( 0 minutes 40.546 seconds)